vue3 腾讯地图拖动标记获取经纬度
时间: 2023-08-07 22:06:43 浏览: 86
你可以使用腾讯地图的 `Marker` 类来创建标记,并且监听 `dragend` 事件来获取标记位置的经纬度信息。具体实现如下:
1. 在 HTML 中添加地图容器和标记容器:
```html
<div id="map"></div>
<div id="marker"></div>
```
2. 在 JavaScript 中创建地图和标记:
```javascript
// 初始化地图
var map = new qq.maps.Map(document.getElementById("map"), {
center: new qq.maps.LatLng(39.916527, 116.397128),
zoom: 13,
});
// 创建标记
var marker = new qq.maps.Marker({
position: map.getCenter(),
draggable: true,
map: map,
});
// 监听标记拖动结束事件
qq.maps.event.addListener(marker, "dragend", function (event) {
// 获取标记位置的经纬度信息
var latLng = marker.getPosition();
console.log("经度:" + latLng.getLng() + ",纬度:" + latLng.getLat());
});
```
这样就可以在地图上拖动标记,并且在控制台中输出标记位置的经纬度信息了。
相关问题
vue3 使用腾讯地图拖动标记获取经纬度详细步骤
好的,下面是详细的步骤:
1. 在 HTML 中地图容器和标记容器:
```html
<template>
<div class="map-container">
<div class="map" ref="map"></div>
<div class="marker" ref="marker"></div>
</div>
</template>
```
2. 在 `setup()` 函数中引入腾讯地图的 API 和样式文件,并创建地图和标记:
```javascript
import { onMounted, ref } from "vue";
export default {
setup() {
// 引入腾讯地图 API 和样式文件
const script = document.createElement("script");
script.type = "text/javascript";
script.src = "http://map.qq.com/api/js?v=2.exp&key=YOUR_KEY_HERE";
script.onload = () => {
// 创建地图和标记
const map = new qq.maps.Map(document.getElementById("map"), {
center: new qq.maps.LatLng(39.916527, 116.397128),
zoom: 13,
});
const marker = new qq.maps.Marker({
position: map.getCenter(),
draggable: true,
map: map,
});
// 监听标记拖动结束事件
qq.maps.event.addListener(marker, "dragend", function (event) {
// 获取标记位置的经纬度信息
const latLng = marker.getPosition();
console.log("经度:" + latLng.getLng() + ",纬度:" + latLng.getLat());
});
};
document.head.appendChild(script);
return {};
},
};
```
3. 在 `mounted()` 函数中获取地图和标记容器的 DOM 节点:
```javascript
import { onMounted, ref } from "vue";
export default {
setup() {
// ...
onMounted(() => {
// 获取地图和标记容器的 DOM 节点
const mapContainer = document.getElementById("map");
const markerContainer = document.getElementById("marker");
// 设置地图和标记容器的宽度和高度
mapContainer.style.width = "100%";
mapContainer.style.height = "400px";
markerContainer.style.width = "32px";
markerContainer.style.height = "32px";
// 将标记容器作为标记的图标
marker.setIcon(
new qq.maps.MarkerImage(
"http://open.map.qq.com/doc/img/n_marker.png",
null,
null,
null,
new qq.maps.Size(32, 32)
)
);
marker.set("offset", new qq.maps.Size(0, 0));
marker.set("iconAnchor", new qq.maps.Point(16, 16));
marker.set("shadow", new qq.maps.MarkerImage(null, null, null, null, new qq.maps.Size(0, 0)));
markerContainer.appendChild(marker.get("container"));
});
return {};
},
};
```
4. 最后在 CSS 中设置地图容器的样式:
```css
.map-container {
position: relative;
}
.map {
position: relative;
z-index: 1;
}
.marker {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
z-index: 2;
}
```
这样就完成了使用腾讯地图拖动标记获取经纬度的操作。
vue2谷歌地图点击地图获取经纬度
在Vue2中,你可以使用谷歌地图的API来实现点击地图获取经纬度的功能。下面是一个简单的示例代码:
首先,在Vue组件中引入Google Maps API的脚本。你可以将以下代码添加到`index.html`文件的`<head>`标签中:
```html
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap" async defer></script>
```
确保将`YOUR_API_KEY`替换为你自己的谷歌地图API密钥。
然后,在你的Vue组件中,你可以创建一个地图并添加一个监听器来获取点击事件的经纬度。以下是示例代码:
```vue
<template>
<div id="map"></div>
</template>
<script>
export default {
mounted() {
// 初始化地图
this.initMap();
},
methods: {
initMap() {
// 创建地图
const map = new google.maps.Map(document.getElementById("map"), {
center: { lat: 0, lng: 0 },
zoom: 8,
});
// 添加点击事件监听器
map.addListener("click", (event) => {
const lat = event.latLng.lat();
const lng = event.latLng.lng();
console.log(`经度:${lng.toFixed(6)}, 纬度:${lat.toFixed(6)}`);
// 在这里可以对获取的经纬度进行处理或发送到后端等操作
});
},
},
};
</script>
```
在上述代码中,我们首先在`mounted`生命周期钩子中调用`initMap`方法来初始化地图。在`initMap`方法中,我们创建了一个新的地图实例,并将其绑定到指定的DOM元素上。然后,我们添加了一个点击事件监听器,并在回调函数中获取了点击位置的经纬度。
请注意,你需要替换示例代码中的`YOUR_API_KEY`为你自己的谷歌地图API密钥。
相关推荐
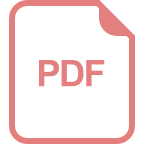
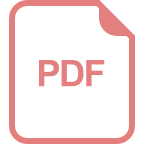












