queue python
时间: 2023-10-14 09:23:59 浏览: 39
A queue in Python is a linear data structure that follows the First-In-First-Out (FIFO) principle. It is similar to a real-life queue, where the first person to enter the queue is the first person to leave it.
In Python, a queue can be implemented using the built-in module 'queue'. There are two types of queues that can be created using this module: the 'Queue' class and the 'PriorityQueue' class.
The 'Queue' class is a basic queue that follows the FIFO principle. It has the following methods:
- put(item): Adds an item to the end of the queue.
- get(): Removes and returns the item at the front of the queue.
- empty(): Returns True if the queue is empty, False otherwise.
- qsize(): Returns the number of items in the queue.
The 'PriorityQueue' class is a queue that orders the items based on their priority. It has the same methods as the 'Queue' class, but it also has a 'put(item, priority)' method that allows the user to specify the priority of the item being added.
Here's an example of how to create and use a 'Queue' object in Python:
```
import queue
q = queue.Queue()
q.put(1)
q.put(2)
q.put(3)
while not q.empty():
print(q.get())
```
Output:
```
1
2
3
```
In this example, we create a 'Queue' object and add three items to it using the 'put' method. We then use a while loop to remove and print each item using the 'get' method. Since the queue follows the FIFO principle, the items are printed in the order they were added.
相关推荐
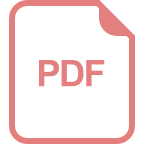
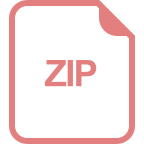
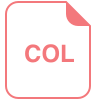











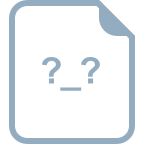
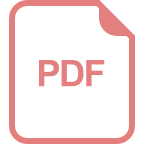
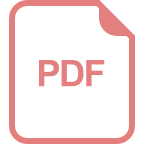
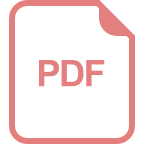