如何判断 PendingIntent是否存在
时间: 2024-03-06 15:51:00 浏览: 219
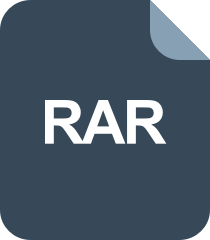
Service实现通知,判断通知栏是否已打开

可以通过以下方式判断 PendingIntent 是否存在:
1. 使用 PendingIntent 的 getActivity()、getService() 或 getBroadcast() 方法获取 PendingIntent 对应的 Intent 对象。
2. 使用 PendingIntent 的 FLAG_NO_CREATE 标志来获取 PendingIntent 对象,如果返回的 PendingIntent 值为 null,则表示 PendingIntent 不存在。
具体代码如下:
```
Intent intent = new Intent(context, YourActivity.class);
PendingIntent pendingIntent = PendingIntent.getActivity(context, requestCode, intent, PendingIntent.FLAG_NO_CREATE);
if (pendingIntent != null) {
// PendingIntent 存在
} else {
// PendingIntent 不存在
}
```
阅读全文
相关推荐
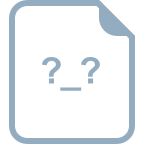
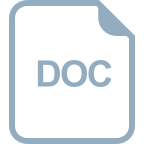





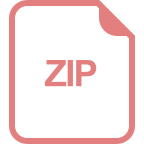
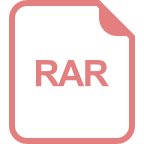
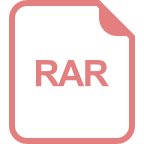
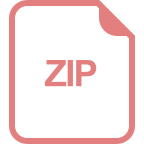
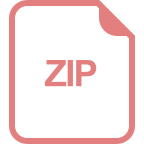
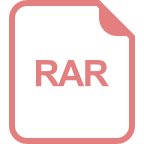
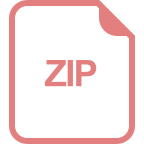
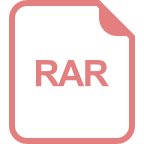