WPF用户控件定义自定义属性,并绑定到对应控件上
时间: 2024-03-03 15:53:22 浏览: 78
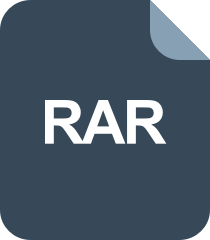
WPF带属性的用户控件

在WPF中,我们可以通过依赖属性(Dependency Property)来定义自定义属性,并绑定到对应的控件上。
以下是一个示例,演示如何定义自定义属性,并绑定到按钮(Button)上:
1. 定义依赖属性
```csharp
public static readonly DependencyProperty MyCustomProperty =
DependencyProperty.Register(
"MyCustom",
typeof(string),
typeof(MyUserControl),
new PropertyMetadata(string.Empty));
public string MyCustom
{
get { return (string)GetValue(MyCustomProperty); }
set { SetValue(MyCustomProperty, value); }
}
```
在上面的代码中,我们使用DependencyProperty.Register方法来定义一个名为MyCustom的依赖属性。该属性的类型为string,所属的控件类型为MyUserControl。我们还通过PropertyMetadata设置了该属性的默认值为string.Empty。最后,我们定义了一个公共属性MyCustom,用于获取或设置该依赖属性的值。
2. 为控件添加自定义属性
```xml
<UserControl x:Class="MyUserControl"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="300">
<Grid>
<Button Content="Click Me" MyCustom="Hello World!" />
</Grid>
</UserControl>
```
在上面的代码中,我们为Button控件添加了MyCustom属性,并设置了该属性的值为"Hello World!"。
3. 绑定自定义属性
```xml
<UserControl x:Class="MyUserControl"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="300">
<Grid>
<Button Content="Click Me" MyCustom="{Binding MyValue}" />
</Grid>
</UserControl>
```
在上面的代码中,我们使用了数据绑定来绑定MyCustom属性。我们将该属性绑定到一个名为MyValue的属性上,这个属性可以是控件的DataContext属性,也可以是控件的父级元素属性。当MyValue属性的值发生变化时,MyCustom属性也会自动更新。
阅读全文
相关推荐
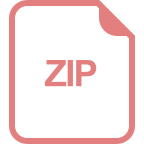
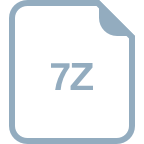
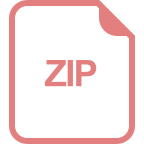
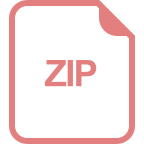
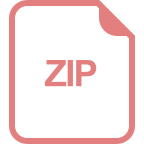
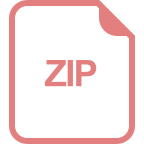
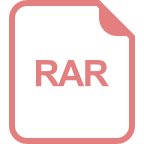
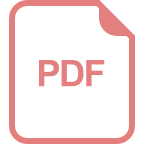
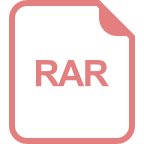
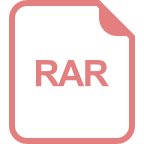
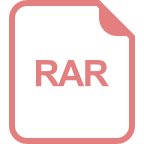
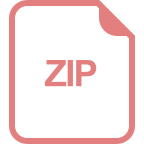
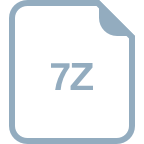
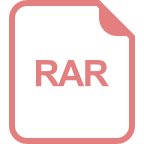
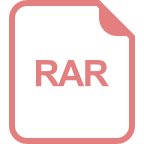
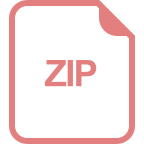