用html,css,Vue3提供代码
时间: 2024-09-30 07:16:19 浏览: 32
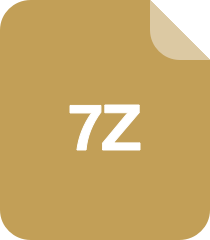
前端面试大全,自己整理
基于您提供的需求描述,这里给出一个简单的HTML+CSS+Vue3的基础示例来实现类似的功能。请注意,这只是一个基础框架,并且为了简化说明省略了一些细节和错误处理部分:
首先,需要引入Vue.js。如果你已经配置了Vue环境,则可以直接编写组件;否则可以通过CDN链接引入Vue运行版(注意要使用生产版本):
```html
<script src="https://cdn.jsdelivr.net/npm/vue@3/dist/vue.global.prod.js"></script>
```
接下来是基本的HTML结构:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
/* 这里放置样式 */
.container {
width: 80%;
margin: auto;
}
form, ul {
list-style-type: none;
padding-left: 0;
}
li {
display: flex;
justify-content: space-between;
align-items: center;
}
</style>
</head>
<body>
<div id="app" class="container">
<!-- 输入框 -->
<form @submit.prevent="addHobby">
<input v-model="newHobby" placeholder="请输入新的爱好..." />
<button type="submit">提交</button>
</form>
<!-- 其他功能如:删除、修改等 -->
<ul>
<li v-for="(hobby, index) in hobbies" :key="index">
{{ hobby }}
<button @click="removeHobby(index)">删除</button>
<!-- 修改按钮等 -->
</li>
</ul>
<!-- 查询功能 -->
<input v-model="searchId" placeholder="按学号搜索...">
<button @click="filterById">查找</button>
<!-- 总分与均分显示 -->
<p>总分: {{ totalScore }}</p>
<p>平均分: {{ averageScore }}</p>
</div>
<script>
// 假设这是个Vue实例
const { createApp } = Vue;
createApp({
data() {
return {
newHobby: '',
searchId: '',
hobbies: ['Reading', 'Coding'], // 示例数据
scores: [90, 80] // 对应的分数
};
},
computed: {
totalScore() {
return this.scores.reduce((acc, score) => acc + score, 0);
},
averageScore() {
if(this.scores.length > 0){
return this.totalScore / this.scores.length;
} else {
return 0;
}
}
},
methods: {
addHobby() {
this.hobbies.push(this.newHobby);
this.newHobby = '';
},
removeHobby(index) {
this.hobbies.splice(index, 1);
this.scores.splice(index, 1); // 同时移除对应的分数
},
filterById() {
const id = parseInt(this.searchId);
this.hobbies = this.hobbies.filter((_, i) => id === i);
this.scores = this.scores.filter((score, i) => id === i);
}
}
}).mount('#app');
</script>
</body>
</html>
```
以上代码片段展示了如何使用Vue 3创建一个应用以管理用户的兴趣爱好。它包含了添加新爱好的表单,以及用于删除已有项目的按钮。同时实现了根据索引模拟“学号”的查询功能,并计算所有条目的总分及平均分。你可以在此基础上进一步完善具体逻辑或扩展其他功能模块。
阅读全文
相关推荐
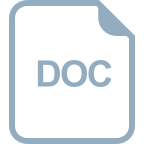
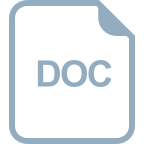
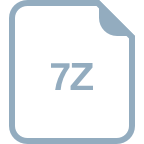
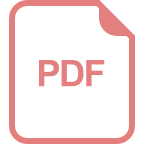
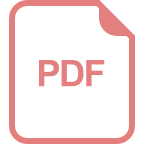
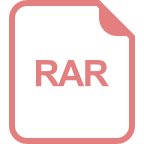
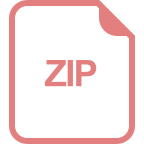
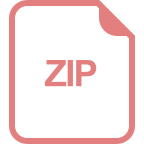
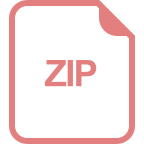
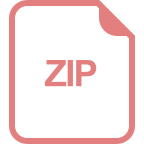
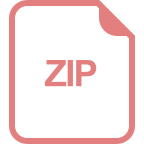
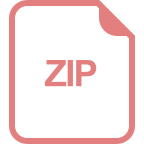
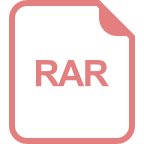
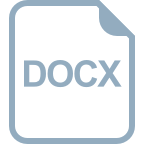
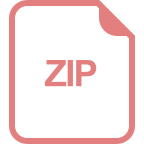
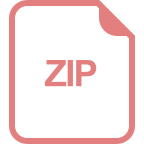
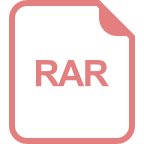
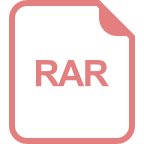