请帮我用verilog语言写出中断优先级排队电路的设计,并详细的解释
时间: 2024-03-06 14:51:28 浏览: 286
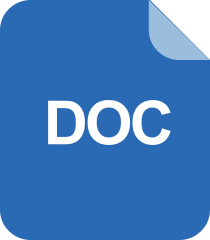
优先权排队电路设计说明.doc
好的,这里是一个简单的中断优先级排队电路的设计示例:
```verilog
module interrupt_queue (
input clk,
input [3:0] interrupt_priority,
input [3:0] interrupt_enable,
input reset,
output [3:0] interrupt_acknowledged
);
reg [3:0] queue [0:15];
reg [3:0] head;
reg [3:0] tail;
always @(posedge clk) begin
if (reset) begin
head <= 4'b0000;
tail <= 4'b0000;
for (int i = 0; i < 16; i = i + 1) begin
queue[i] <= 4'b0000;
end
end else if (interrupt_enable != 4'b0000) begin
queue[tail] <= interrupt_priority;
tail <= tail + 1;
if (tail == 4'b10000) begin
tail <= 4'b0000;
end
if (head == tail) begin
head <= head + 1;
if (head == 4'b10000) begin
head <= 4'b0000;
end
end
end
end
assign interrupt_acknowledged = queue[head];
endmodule
```
这个设计包括一个 4 位的中断优先级输入 `interrupt_priority`,一个 4 位的中断使能输入 `interrupt_enable`,一个时钟输入 `clk`,一个复位输入 `reset`,和一个 4 位的中断确认输出 `interrupt_acknowledged`。
这个设计使用一个环形缓冲区来存储中断。当 `interrupt_enable` 的某一位为 1 时,表示对应的中断被触发,此时将对应的 `interrupt_priority` 值存入队列中。队列使用环形缓冲区实现,当队列满时,新的中断将覆盖队列头上的中断。中断确认输出为队列头上的中断优先级。
下面逐行解释代码:
```verilog
module interrupt_queue (
input clk,
input [3:0] interrupt_priority,
input [3:0] interrupt_enable,
input reset,
output [3:0] interrupt_acknowledged
);
```
这是一个模块定义,包括一个时钟输入 `clk`,一个 4 位的中断优先级输入 `interrupt_priority`,一个 4 位的中断使能输入 `interrupt_enable`,一个复位输入 `reset`,和一个 4 位的中断确认输出 `interrupt_acknowledged`。
```verilog
reg [3:0] queue [0:15];
```
这是一个大小为 16 的 4 位寄存器数组,用于存储中断队列。
```verilog
reg [3:0] head;
reg [3:0] tail;
```
这是两个 4 位寄存器,用于记录队列头和队列尾的位置。
```verilog
always @(posedge clk) begin
```
这是一个时钟边沿触发的 `always` 块。
```verilog
if (reset) begin
head <= 4'b0000;
tail <= 4'b0000;
for (int i = 0; i < 16; i = i + 1) begin
queue[i] <= 4'b0000;
end
end
```
如果复位信号 `reset` 为 1,那么将队列头和队列尾的位置重置为 0,同时清空队列中的所有中断。
```verilog
else if (interrupt_enable != 4'b0000) begin
queue[tail] <= interrupt_priority;
tail <= tail + 1;
if (tail == 4'b10000) begin
tail <= 4'b0000;
end
if (head == tail) begin
head <= head + 1;
if (head == 4'b10000) begin
head <= 4'b0000;
end
end
end
```
如果有中断被触发,即 `interrupt_enable` 中的某一位为 1,那么将对应的中断优先级值存入队列中。队列尾指针 `tail` 加 1,如果 `tail` 达到队列的末尾,则将其重置为 0。如果队列已满(即 `head` 和 `tail` 的值相等),则将队列头指针 `head` 加 1,并将其重置为 0 如果 `head` 达到队列的末尾。
```verilog
assign interrupt_acknowledged = queue[head];
```
将队列头上的中断优先级赋给中断确认输出。
希望这样的解释对您有所帮助。
阅读全文
相关推荐
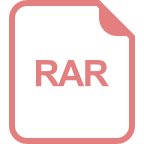
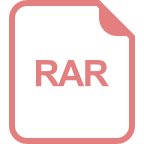






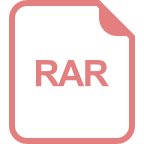
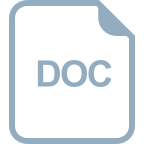





