使用C语言编写程序实现栈和队列的实现和应用,完整代码
时间: 2025-01-05 10:40:23 浏览: 17
在C语言中,栈和队列是基础的数据结构,可以手动用数组或链表来实现。这里我会分别给出简单的栈和队列的实现示例以及它们的一些基本操作。
**栈(Stack)实现:**
```c
#include <stdio.h>
#define MAX_SIZE 50
typedef struct {
int top;
int data[MAX_SIZE];
} Stack;
void push(Stack *stack, int item) {
if (stack->top == MAX_SIZE - 1) {
printf("Stack is full.\n");
} else {
stack->data[++stack->top] = item;
printf("Pushed %d onto the stack.\n", item);
}
}
int pop(Stack *stack) {
if (stack->top == -1) {
printf("Stack is empty.\n");
return -1; // 返回-1表示空栈
} else {
return stack->data[stack->top--];
}
}
int main() {
Stack s;
s.top = -1;
push(&s, 10);
push(&s, 20);
printf("Top element is: %d\n", pop(&s));
return 0;
}
```
**队列(Queue)实现:**
```c
#include <stdio.h>
#define MAX_SIZE 50
typedef struct {
int front;
int rear;
int data[MAX_SIZE];
} Queue;
void enqueue(Queue *queue, int item) {
if (queue- 1) {
printf("Queue is full.\n");
} else {
queue->data[++queue->rear] = item;
printf("Enqueued %d into the queue.\n", item);
}
}
int dequeue(Queue *queue) {
if (queue->front == queue->rear) {
printf("Queue is empty.\n");
return -1; // 空队列处理
} else {
int removed_item = queue->data[queue->front++];
return removed_item;
}
}
int main() {
Queue q;
q.front = q.rear = -1;
enqueue(&q, 1);
enqueue(&q, 2);
printf("Dequeued item is: %d\n", dequeue(&q));
return 0;
}
```
阅读全文
相关推荐



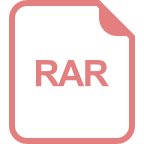




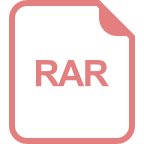









