#include <iostream> using namespace std; class IndexError{}; template class ARRAY { size_t m_size; T *m_ptr; public: ARRAY(size_t size) : m_size(size) { m_ptr = new T[size]; memset(m_ptr, 0, size*sizeof(int)); } ~ARRAY() { delete[] m_ptr; } T& at(int index); }; template <typename T> ::at(int index) { if(index<0|| ) { IndexError(); } return m_ptr[index]; } int main() { ARRAY<int> a(50); int i; cin >> i; { for(int j=0;j<i;j++) a.at(i) = j; } catch(IndexError e) { return 0; } return 0; }
时间: 2024-01-10 22:04:15 浏览: 213
这段代码有一些问题,我来帮您指出:
1. 在模板类 `ARRAY` 的函数 `at` 中,没有指定返回类型。
2. 在 `at` 函数中,如果下标越界,应该抛出异常,但是现在抛出异常的方式是通过调用一个函数 `IndexError()`,这是错误的。正确的方式应该是使用 `throw` 抛出一个异常对象。
3. 在 `main` 函数中,`for` 循环中的下标应该从 0 开始,而不是从 `i` 开始,这样才能正确地给数组赋值。
下面是修改后的代码:
```c++
#include <iostream>
#include <cstring>
using namespace std;
class IndexError{};
template <typename T>
class ARRAY {
size_t m_size;
T *m_ptr;
public:
ARRAY(size_t size) : m_size(size) {
m_ptr = new T[size];
memset(m_ptr, 0, size*sizeof(T));
}
~ARRAY() {
delete[] m_ptr;
}
T& at(int index) {
if (index < 0 || index >= m_size) {
throw IndexError();
}
return m_ptr[index];
}
};
int main() {
int i;
cin >> i;
try {
ARRAY<int> a(i);
for (int j = 0; j < i; j++) {
a.at(j) = j;
}
}
catch (IndexError e) {
return 0;
}
return 0;
}
```
这样就可以正确地创建一个 `ARRAY` 对象并设置其中的元素了。如果下标越界,会抛出 `IndexError` 异常。
相关推荐
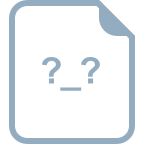
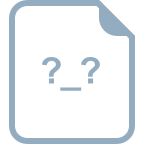















