用Java写出来两个数组匹配第一个相同值的kmp算法
时间: 2023-07-20 16:15:20 浏览: 75
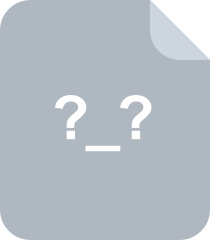
java实现的kmp算法

以下是用Java实现的KMP算法,用于匹配两个数组中第一个相同的值:
```java
public class KMP {
public static int findFirstMatch(int[] array1, int[] array2) {
int[] prefix = computePrefix(array2);
int j = 0;
for (int i = 0; i < array1.length; i++) {
while (j > 0 && array1[i] != array2[j]) {
j = prefix[j - 1];
}
if (array1[i] == array2[j]) {
j++;
}
if (j == array2.length) {
return i - j + 1;
}
}
return -1;
}
private static int[] computePrefix(int[] array) {
int[] prefix = new int[array.length];
int j = 0;
for (int i = 1; i < array.length; i++) {
while (j > 0 && array[i] != array[j]) {
j = prefix[j - 1];
}
if (array[i] == array[j]) {
j++;
}
prefix[i] = j;
}
return prefix;
}
public static void main(String[] args) {
int[] array1 = {1, 2, 3, 4, 5};
int[] array2 = {6, 7, 8, 4, 5};
int index = findFirstMatch(array1, array2);
if (index != -1) {
System.out.println("The first matching index is: " + index);
} else {
System.out.println("No matching index is found.");
}
}
}
```
这里我们定义了一个 `findFirstMatch` 方法,用来查找两个数组中第一个相同的值。我们首先计算出 `array2` 的前缀数组 `prefix`,然后使用两个指针 `i` 和 `j` 分别指向 `array1` 和 `array2` 的起始位置,如果 `array1[i]` 和 `array2[j]` 相等,则将 `i` 和 `j` 都向后移动一位,如果 `array1[i]` 和 `array2[j]` 不相等,则将 `j` 移动到 `prefix[j-1]` 的位置,然后再继续比较。如果 `j` 移动到了 `array2` 的末尾,则说明找到了第一个匹配的位置,返回该位置即可。如果最终没有找到匹配的位置,则返回 `-1`。
阅读全文
相关推荐
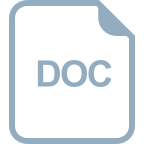
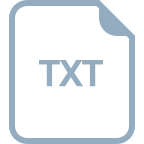
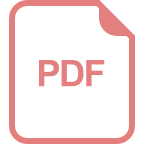
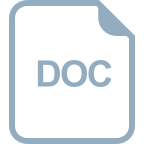
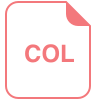
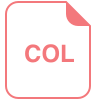



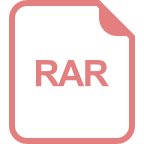
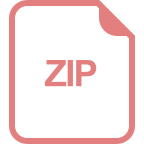
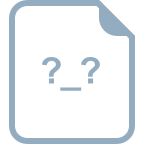
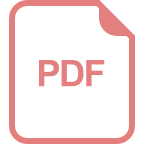
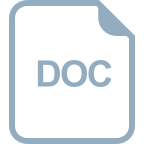
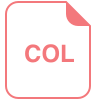
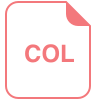