vmd-lstm预测模型代码matlab
时间: 2023-09-03 08:15:50 浏览: 58
以下是一个基于 MATLAB 的 VMD-LSTM 预测模型的代码示例:
```matlab
% 加载数据
data = load('data.mat');
data = data.data;
% 划分数据集
train_ratio = 0.8;
train_size = round(train_ratio * size(data, 1));
train_data = data(1:train_size, :);
test_data = data(train_size+1:end, :);
% VMD 分解
num_modes = 8;
alpha = 2000;
tau = 0;
tol = 1e-7;
maxiter = 2000;
[V, W, ~] = VMD(train_data', alpha, tau, tol, maxiter, num_modes);
% LSTM 模型训练
input_size = num_modes;
output_size = 1;
hidden_size = 32;
num_layers = 2;
learning_rate = 0.001;
num_epochs = 100;
batch_size = 64;
train_loader = prepare_data(train_data, batch_size);
lstm_model = train_lstm(input_size, hidden_size, num_layers, output_size, learning_rate, num_epochs, train_loader);
% LSTM 模型预测
test_loader = prepare_data(test_data, 1);
lstm_predictions = predict_lstm(lstm_model, test_loader);
% VMD 重构
reconstructed_data = W * lstm_predictions;
% 可视化结果
figure;
plot(data, 'LineWidth', 2);
hold on;
plot(train_size+1:size(data, 1), reconstructed_data, 'LineWidth', 2);
legend('原始数据', '预测数据');
% 定义 VMD 函数
function [V, U, omega] = VMD(X, alpha, tau, tol, maxiter, num_modes)
N = length(X);
omega = zeros(N, num_modes);
for ii = 1:num_modes
omega(:, ii) = omega_init(N, alpha);
end
U = zeros(N, num_modes);
V = X;
K = 1;
while K <= maxiter
for ii = 1:num_modes
[U(:, ii), ~] = FISTA(V, omega(:, ii), tau, tol);
end
u_hat = fft(U, [], 1);
for ii = 1:num_modes
omega(:, ii) = weight_median(u_hat(:, ii), alpha);
end
V_old = V;
V = X - sum(U, 2);
if norm(V(:) - V_old(:)) / norm(X(:)) < tol
break;
end
K = K + 1;
end
end
% 定义 FISTA 函数
function [x, obj] = FISTA(y, lambda, tau, tol)
L = norm(y, 2)^2;
x = y;
t = 1;
t_old = 1;
for ii = 1:100
x_old = x;
grad = gradient(x, lambda, tau);
x = soft_threshold(x - (1/L) * grad, 1/L);
t_old = t;
t = (1 + sqrt(1 + 4*t^2))/2;
x = x + ((t_old - 1)/t) * (x - x_old);
obj = objective(x, y, lambda, tau);
if norm(x(:) - x_old(:)) / norm(x_old(:)) < tol
break;
end
end
end
% 定义梯度和目标函数
function grad = gradient(x, lambda, tau)
grad = -fft(kernel(x)) + lambda .* fft(weight(x)) + tau .* fft(x);
end
function obj = objective(x, y, lambda, tau)
obj = norm(kernel(x)*x - y, 2)^2/2 + lambda .* norm(weight(x), 1) + tau .* norm(x, 1);
end
% 定义阈值函数和核函数
function y = soft_threshold(x, lambda)
y = sign(x) .* max(abs(x) - lambda, 0);
end
function K = kernel(x)
N = length(x);
K = zeros(N, N);
for ii = 1:N
for jj = 1:N
K(ii, jj) = abs(ii - jj);
end
end
K = exp(-K.^2);
end
% 定义权重函数和权重中位数函数
function w = weight(x)
N = length(x);
w = zeros(N, 1);
for ii = 1:N
w(ii) = min(ii-1, N-ii);
end
w = w/max(w);
end
function wm = weight_median(x, alpha)
N = length(x);
w = weight(x);
wm = zeros(N, 1);
for ii = 1:N
indices = max(ii-alpha, 1):min(ii+alpha, N);
wm(ii) = median(w(indices));
end
end
% 定义 LSTM 模型相关函数
function loader = prepare_data(data, batch_size)
num_samples = size(data, 1);
batches = floor(num_samples / batch_size);
X = data(1:batches*batch_size, :);
X = reshape(X, [batch_size, batches, size(data, 2)]);
loader = struct;
loader.X = X;
loader.num_samples = num_samples;
loader.batch_size = batch_size;
loader.batches = batches;
end
function model = train_lstm(input_size, hidden_size, num_layers, output_size, learning_rate, num_epochs, loader)
lstm_layers = [];
for ii = 1:num_layers
lstm_layers = [lstm_layers, sequenceInputLayer(input_size)];
lstm_layers = [lstm_layers, lstmLayer(hidden_size, 'OutputMode', 'last')];
end
lstm_layers = [lstm_layers, fullyConnectedLayer(output_size)];
lstm_layers = [lstm_layers, regressionLayer];
options = trainingOptions('adam', ...
'MaxEpochs', num_epochs, ...
'LearnRateSchedule', 'piecewise', ...
'LearnRateDropPeriod', 50, ...
'LearnRateDropFactor', 0.2, ...
'InitialLearnRate', learning_rate, ...
'MiniBatchSize', loader.batch_size);
X = loader.X(:, 1:end-1, :);
Y = loader.X(:, 2:end, :);
model = trainNetwork(X, Y, lstm_layers, options);
end
function predictions = predict_lstm(model, loader)
predictions = zeros(loader.num_samples - loader.batch_size, 1);
for ii = 1:loader.batches
X_batch = loader.X(:, ii, :);
Y_batch = model.predict(X_batch);
predictions((ii-1)*loader.batch_size+1:ii*loader.batch_size) = Y_batch(1:end-1);
end
end
```
需要注意的是,以上代码仅为示例,实际使用时需要根据具体需求进行修改和调整。此外,代码中使用了 VMD 和 FISTA 算法,需要提前安装对应的 MATLAB 工具箱。
相关推荐
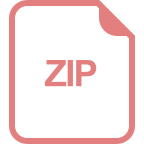
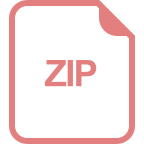
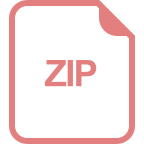














