从二进制文件中读取学生信息包括学号,课程名,考试成绩,将课程名为C的记录按照成绩升序排列,排序后的结果写入文本文件 点击复制后,将打开C知道体验页用C语言
时间: 2023-05-20 07:03:58 浏览: 95
可以使用以下代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct {
int id;
char course[20];
int score;
} Student;
int cmp(const void *a, const void *b) {
Student *s1 = (Student *)a;
Student *s2 = (Student *)b;
if (strcmp(s1->course, s2->course) == 0) {
return s1->score - s2->score;
}
return strcmp(s1->course, s2->course);
}
int main() {
FILE *fp = fopen("students.bin", "rb");
if (fp == NULL) {
printf("Failed to open file.\n");
return 1;
}
fseek(fp, 0, SEEK_END);
int size = ftell(fp);
rewind(fp);
int n = size / sizeof(Student);
Student *students = (Student *)malloc(n * sizeof(Student));
fread(students, sizeof(Student), n, fp);
fclose(fp);
qsort(students, n, sizeof(Student), cmp);
fp = fopen("result.txt", "w");
if (fp == NULL) {
printf("Failed to create file.\n");
return 1;
}
for (int i = 0; i < n; i++) {
if (strcmp(students[i].course, "C") == 0) {
fprintf(fp, "%d %s %d\n", students[i].id, students[i].course, students[i].score);
}
}
fclose(fp);
free(students);
return 0;
}
```
这段代码首先打开二进制文件,读取学生信息并存储在一个结构体数组中。然后使用 `qsort` 函数按照课程名和成绩排序。最后将课程名为 C 的记录写入文本文件中。
阅读全文
相关推荐
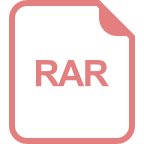
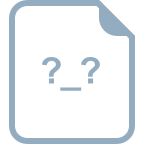
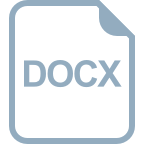
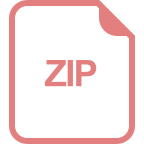
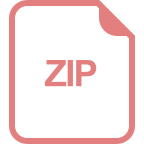