我想通过添加按钮的方式,使用按钮打开子页面,即可用字母“h”打开和关闭,也可使用鼠标点击打开或开闭
时间: 2024-03-22 13:40:04 浏览: 56
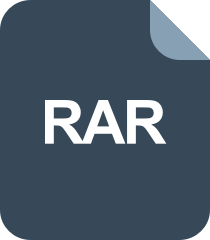
控制页面展开和关闭。
好的,下面是一个示例代码,它可以通过添加按钮的方式,使用按钮打开子页面,也可以使用鼠标点击打开或关闭:
```python
import pygame
# 初始化Pygame
pygame.init()
# 创建游戏窗口
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("My Game")
# 设置字体和字号
font = pygame.font.Font(None, 24)
# 创建游戏规则说明
rule_text = "Welcome to My Game! Press 'h' or click the button to view the game rules."
# 创建按钮
button_width = 100
button_height = 50
button_position = ((screen_width - button_width) // 2, (screen_height - button_height) // 2)
button_color = (255, 255, 255)
button_text = "Rules"
button_font = pygame.font.Font(None, 24)
button_text_color = (0, 0, 0)
button = pygame.Surface((button_width, button_height))
button.fill(button_color)
button_rect = button.get_rect()
button_rect.topleft = button_position
button_text_surface = button_font.render(button_text, True, button_text_color)
button_text_rect = button_text_surface.get_rect()
button_text_rect.center = button_rect.center
button.blit(button_text_surface, button_text_rect)
# 创建文本框
text_box_width = 400
text_box_height = 100
text_box_color = (255, 255, 255)
text_box = pygame.Surface((text_box_width, text_box_height))
text_box.fill(text_box_color)
# 更新文本框内容
rule_surface = font.render(rule_text, True, (0, 0, 0))
text_box.blit(rule_surface, (10, 10))
# 游戏循环
running = True
show_rules = False
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_h:
# 切换显示游戏规则说明
show_rules = not show_rules
elif event.key == pygame.K_ESCAPE:
# 关闭游戏规则说明
show_rules = False
elif event.type == pygame.MOUSEBUTTONDOWN:
if button_rect.collidepoint(event.pos):
# 切换显示游戏规则说明
show_rules = not show_rules
# 绘制屏幕
screen.fill((0, 0, 0))
if show_rules:
screen.blit(text_box, ((screen_width - text_box_width) // 2, (screen_height - text_box_height) // 2))
else:
screen.blit(button, button_rect)
# 更新屏幕
pygame.display.flip()
# 退出Pygame
pygame.quit()
```
在这个示例代码中,我们首先创建了一个游戏窗口,并且设置了窗口标题。然后,我们创建了一个按钮,并且使用Pygame中的Surface对象和字体库设置了按钮的颜色、文本、位置和字号。接着,我们设置了游戏规则说明,并且使用Pygame中的Surface对象在文本框中显示了游戏规则说明。在游戏循环中,我们监听了键盘事件和鼠标事件,如果玩家按下了'h'键或者点击了按钮,就会在屏幕中心显示游戏规则说明。如果玩家按下了'Esc'键或者点击了屏幕其他位置,就会关闭游戏规则说明。最后,我们在每次循环结束后更新了屏幕。
阅读全文
相关推荐
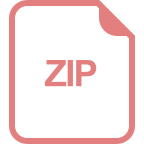
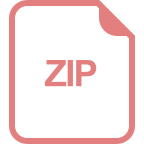
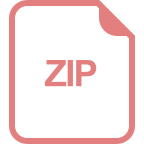
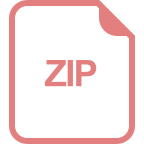
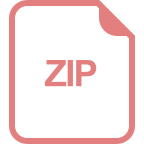
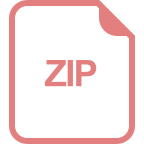
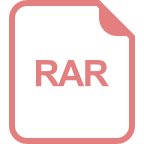
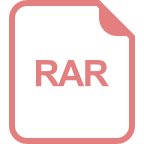
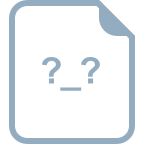
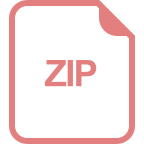
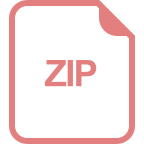
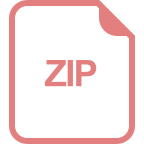
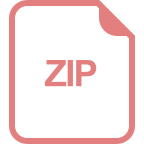