展示lineGroupClearAndInit方法后的代码转换为vue3
时间: 2024-12-08 15:15:50 浏览: 6
将 `lineGroupClearAndInit` 方法及其后续代码从 Vue 2 转换为 Vue 3,主要涉及以下几点:
1. **生命周期钩子**:Vue 3 使用 Composition API 或改进的 Options API 来管理生命周期钩子。
2. **引用和响应式**:使用 `ref` 和 `reactive` 替代 Vue 2 的 `$data`。
3. **模板引用**:使用 `ref` 来获取 DOM 元素或组件实例。
以下是转换后的代码示例:
### 前提条件
假设我们已经引入了必要的 Vue 3 依赖项和地图库,例如 Leaflet 和 AMap。
### 代码转换
#### 1. 引入依赖
```javascript
import { ref, onMounted } from 'vue';
import L from 'leaflet';
import store from '@/store/';
import { getAction } from '@/api/manage';
import { gcoordTransform } from '@/utils/gcoordTransform';
import AMapLoader from '@amap/amap-jsapi-loader';
```
#### 2. 定义组件
```javascript
export default {
setup() {
const leafletMap = ref(null);
const linesGroup = ref([]);
const linesDirectionGroup = ref([]);
const lineList = ref([]);
const currentPipelinetId = ref('');
const pipelinePosLen = ref(0);
const allLineList = ref([]);
// 其他数据...
const resizeFlag = ref(false);
const ciLayer = ref(null);
// 定义 lineGroupClearAndInit 方法
const lineGroupClearAndInit = () => {
// 移除 linesGroup 中的所有层
linesGroup.value.forEach(layer => {
leafletMap.value.removeLayer(layer);
});
// 清空 linesGroup 数组
linesGroup.value = [];
// 移除 linesDirectionGroup 中的所有层
linesDirectionGroup.value.forEach(layer => {
leafletMap.value.removeLayer(layer);
});
// 遍历 lineList 数组,并重置 'operation' 属性和更新 'weight'
lineList.value.forEach(item => {
if (item.operation === 'edit') {
item.operation = '';
item.weight = 2;
}
});
// 修改数据
if (currentPipelinetId.value) {
// 点击的管段
if (pipelinePosLen.value === 4) {
const index = allLineList.value.findIndex(item => item.id === currentPipelinetId.value);
if (index !== -1) {
lineList.value[index].operation = 'edit';
lineList.value[index].weight = 6;
} else {
const newItem = allLineList.value.find(item => item.id === currentPipelinetId.value);
if (newItem) {
newItem.operation = 'add';
lineList.value.push(newItem);
}
}
}
// 点击的管线
if (pipelinePosLen.value === 3) {
lineList.value.forEach(item => {
if (item.superiorPipelineId === currentPipelinetId.value) {
item.operation = 'edit';
item.weight = 6;
}
});
}
}
};
// 获取地图
const getMap = () => {
leafletMap.value = new L.Map('mapid', {
zoom: 14,
center: store.getters.gisMapCenter,
boxZoom: true,
zoomControl: false,
doubleClickZoom: false,
attributionControl: false,
preferCanvas: true
});
leafletMap.value.on('zoomend', function (e) {
// 处理缩放结束后移除和添加标记的逻辑
});
const layer = L.tileLayer('//webrd0{s}.is.autonavi.com/appmaptile?lang=zh_cn&size=1&scale=1&style=8&x={x}&y={y}&z={z}', {
maxZoom: 20,
maxNativeZoom: 18,
minZoom: 3,
attribution: "高德地图 AutoNavi.com",
subdomains: "1234"
}).addTo(leafletMap.value);
L.control.zoom({
zoomInTitle: '放大',
zoomOutTitle: '缩小',
position: 'bottomright'
}).addTo(leafletMap.value);
AMapLoader.load({
key: 'your_amap_key', // 替换为你自己的高德地图 Key
version: '2.0',
plugins: []
}).then(AMap => {
const autoOptions = {
input: "addrInput"
};
const auto = new AMap.Autocomplete(autoOptions);
new AMap.PlaceSearch({
map: leafletMap.value
});
AMap.event.addListener(auto, "select", async (e) => {
if (e.poi.location) {
const latlng = [e.poi.location.lat, e.poi.location.lng];
leafletMap.value.setView(latlng);
const marker = L.marker(latlng, {
icon: locationIcon
});
if (leafletMap.value.hasLayer(addrMarkerGroup.value)) {
leafletMap.value.removeLayer(addrMarkerGroup.value);
}
addrMarkerGroup.value = L.layerGroup([marker]);
leafletMap.value.addLayer(addrMarkerGroup.value);
} else {
console.warn('您输入的内容查询不到相关地址!');
}
});
});
};
// 生命周期钩子
onMounted(() => {
getMap();
});
// 返回可绑定的属性和方法
return {
leafletMap,
lineGroupClearAndInit
};
}
};
```
### 主要变化解释
1. **引用和响应式**:使用 `ref` 和 `reactive` 来创建响应式变量。
2. **方法定义**:在 `setup` 函数内部定义方法。
3. **生命周期钩子**:使用 `onMounted` 来替代 Vue 2 的 `mounted` 钩子。
4. **模板引用**:如果需要访问 DOM 元素,可以使用 `ref` 进行模板引用。
这样,我们将 Vue 2 项目中的方法成功转换为 Vue 3 的 Composition API 风格。
阅读全文
相关推荐
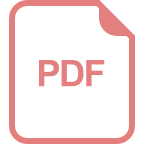
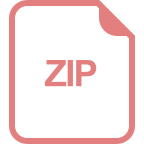
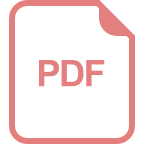















