用C语言写一段本题要求实现一个计算复数之和的简单函数。
时间: 2024-03-08 10:47:16 浏览: 24
好的,以下是用 C 语言实现计算两个复数之和的函数:
```c
#include <stdio.h>
typedef struct {
double real;
double imag;
} complex;
complex complex_addition(complex c1, complex c2) {
complex result;
result.real = c1.real + c2.real;
result.imag = c1.imag + c2.imag;
return result;
}
int main() {
complex c1 = {1.0, 2.0};
complex c2 = {3.0, 4.0};
complex sum = complex_addition(c1, c2);
printf("The sum of two complex numbers is: %f + %fi\n", sum.real, sum.imag);
return 0;
}
```
在这个程序中,我们首先定义了一个 `complex` 结构体,包含实部和虚部两个成员变量。然后定义了一个 `complex_addition` 函数,用于计算两个复数之和,并返回一个 `complex` 类型的结果。
在 `main` 函数中,我们定义了两个复数 `c1` 和 `c2`,并调用 `complex_addition` 函数计算它们的和。最后,我们将结果打印输出。
需要注意的是,C 语言并没有内置的复数类型,因此我们需要自己定义一个 `complex` 结构体来表示复数。
相关推荐
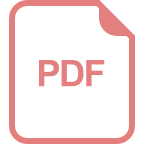
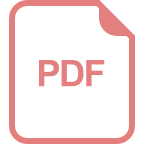
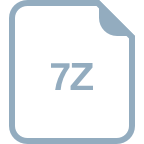















