springboot给属性设置默认值
时间: 2023-10-14 09:28:43 浏览: 313
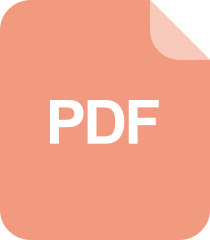
spring boot 中设置默认网页的方法
在Spring Boot中,可以使用@Value注解来设置属性的默认值。例如,假设有一个属性名为my.property,并且需要将其默认值设置为10,可以在应用程序的配置文件(例如application.properties或application.yml)中添加以下内容:
application.properties:
```properties
my.property=10
```
或者使用yml格式:
application.yml:
```yaml
my:
property: 10
```
然后,在类中使用@Value注解将该属性注入到类中:
```java
@Component
public class MyComponent {
@Value("${my.property:5}")
private int myProperty;
// ...
}
```
在上述代码中,@Value注解中的“${my.property:5}”表示从应用程序的配置文件中获取属性my.property的值。如果该属性不存在,则使用默认值5。
阅读全文
相关推荐
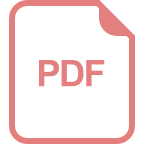





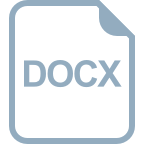









