C语言利用头插法建立一个单链表,随机产生10个100以内的整数,并按要求完成:(1)在屏幕上显示单链表中的10个整数;(2)删除值为a的结点,若不存在a,则把a插入到第i个位置,显示更新后的单链表;
时间: 2024-02-21 15:02:29 浏览: 20
好的,根据您的要求,我给您提供一个C语言的程序,如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
struct ListNode {
int val;
struct ListNode* next;
};
void insert(struct ListNode** head, int val) {
struct ListNode* node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->val = val;
node->next = *head;
*head = node;
}
void printList(struct ListNode* head) {
while (head) {
printf("%d ", head->val);
head = head->next;
}
printf("\n");
}
void deleteNode(struct ListNode** head, int val) {
if (!*head) {
return;
}
if ((*head)->val == val) {
struct ListNode* tmp = *head;
*head = (*head)->next;
free(tmp);
return;
}
struct ListNode* p = *head;
while (p->next) {
if (p->next->val == val) {
struct ListNode* tmp = p->next;
p->next = tmp->next;
free(tmp);
return;
}
p = p->next;
}
}
void insertNode(struct ListNode** head, int i, int val) {
if (i == 0) {
struct ListNode* node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->val = val;
node->next = *head;
*head = node;
return;
}
struct ListNode* p = *head;
while (i > 1 && p) {
p = p->next;
i--;
}
if (!p) {
return;
}
struct ListNode* node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->val = val;
node->next = p->next;
p->next = node;
}
int main() {
srand(time(NULL));
struct ListNode* head = NULL;
for (int i = 0; i < 10; i++) {
int val = rand() % 100 + 1;
insert(&head, val);
}
printf("Original list: ");
printList(head);
int a;
printf("Enter a value to delete or insert: ");
scanf("%d", &a);
if (head && head->val == a) {
struct ListNode* tmp = head;
head = head->next;
free(tmp);
} else {
deleteNode(&head, a);
}
if (head) {
printList(head);
} else {
printf("The list is empty.\n");
}
int i;
printf("Enter a position to insert (1-10): ");
scanf("%d", &i);
if (i > 10 || i < 1) {
printf("Invalid position.\n");
return 0;
}
printf("Enter a value to insert: ");
scanf("%d", &a);
insertNode(&head, i-1, a);
printList(head);
return 0;
}
```
该程序利用头插法建立一个单链表,随机产生10个100以内的整数,并按要求完成:(1)在屏幕上显示单链表中的10个整数;(2)删除值为a的结点,若不存在a,则把a插入到第i个位置,显示更新后的单链表。
相关推荐
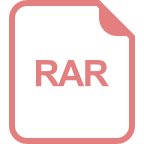














