import org.springframework.beans.factory.annotation.Autowired; import org.springframework.http.ResponseEntity; import org.springframework.web.bind.annotation.*; import java.util.List; @RestController @RequestMapping("/transportation") public class TransportationController<TransportationRepository> { @Autowired private TransportationRepository transportationRepository; @PostMapping("/create") public ResponseEntity<?> createTransportation(@RequestBody TransportationDto transportationDto) { Transportation transportation = new Transportation(); transportation.setFrom(transportationDto.getFrom()); transportation.setTo(transportationDto.getTo()); transportation.setDepartureTime(transportationDto.getDepartureTime()); transportation.setArrivalTime(transportationDto.getArrivalTime()); transportationRepository.save(transportation); return ResponseEntity.ok("Transportation created successfully"); } @GetMapping("/all") public ResponseEntity<?> getAllTransportations() { List<Transportation> transportations = transportationRepository.findAll(); return ResponseEntity.ok(transportations); } @GetMapping("/{id}") public ResponseEntity<?> getTransportationById(@PathVariable Long id) { Transportation transportation = transportationRepository.findById(id).orElse(null); if (transportation == null) { return ResponseEntity.notFound().build(); } return ResponseEntity.ok(transportation); } }这段代码中所有transportationDto的方法报错,比如Cannot resolve method 'getFrom' in 'TransportationDto',请帮我修改
时间: 2024-02-13 13:02:21 浏览: 29
根据报错信息来看,TransportationDto中缺少getFrom等方法,所以需要在TransportationDto中添加相应的getter方法,例如:
```
public class TransportationDto {
private String from;
private String to;
private LocalDateTime departureTime;
private LocalDateTime arrivalTime;
public String getFrom() {
return from;
}
public void setFrom(String from) {
this.from = from;
}
public String getTo() {
return to;
}
public void setTo(String to) {
this.to = to;
}
public LocalDateTime getDepartureTime() {
return departureTime;
}
public void setDepartureTime(LocalDateTime departureTime) {
this.departureTime = departureTime;
}
public LocalDateTime getArrivalTime() {
return arrivalTime;
}
public void setArrivalTime(LocalDateTime arrivalTime) {
this.arrivalTime = arrivalTime;
}
}
```
同时,TransportationController中依赖的TransportationRepository需要声明泛型类型,例如:
```
@RestController
@RequestMapping("/transportation")
public class TransportationController {
@Autowired
private TransportationRepository transportationRepository;
@PostMapping("/create")
public ResponseEntity<?> createTransportation(@RequestBody TransportationDto transportationDto) {
Transportation transportation = new Transportation();
transportation.setFrom(transportationDto.getFrom());
transportation.setTo(transportationDto.getTo());
transportation.setDepartureTime(transportationDto.getDepartureTime());
transportation.setArrivalTime(transportationDto.getArrivalTime());
transportationRepository.save(transportation);
return ResponseEntity.ok("Transportation created successfully");
}
@GetMapping("/all")
public ResponseEntity<?> getAllTransportations() {
List<Transportation> transportations = transportationRepository.findAll();
return ResponseEntity.ok(transportations);
}
@GetMapping("/{id}")
public ResponseEntity<?> getTransportationById(@PathVariable Long id) {
Transportation transportation = transportationRepository.findById(id).orElse(null);
if (transportation == null) {
return ResponseEntity.notFound().build();
}
return ResponseEntity.ok(transportation);
}
}
```
希望这能够帮助到你。
相关推荐
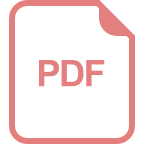
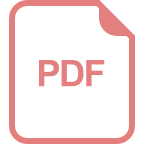
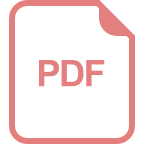















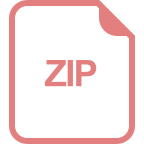