Java Swing 实现字母连连看小游戏的代码
时间: 2023-11-13 14:04:40 浏览: 44
以下是一个简单的 Java Swing 实现字母连连看小游戏的代码:
```java
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
public class LetterLinkGame extends JFrame {
private static final long serialVersionUID = 1L;
private static final int ROWS = 6;
private static final int COLS = 9;
private static final int BUTTON_WIDTH = 60;
private static final int BUTTON_HEIGHT = 60;
private static final String[] LETTERS = {"A", "B", "C", "D", "E", "F", "G", "H", "I", "J"};
private JButton[][] buttons;
private List<String> letters;
private JButton selectedButton;
private int movesLeft;
private int score;
public LetterLinkGame() {
setTitle("Letter Link Game");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setResizable(false);
letters = new ArrayList<>();
for (int i = 0; i < ROWS * COLS / 2; i++) {
letters.add(LETTERS[i]);
letters.add(LETTERS[i]);
}
Collections.shuffle(letters);
JPanel mainPanel = new JPanel(new GridLayout(ROWS, COLS));
buttons = new JButton[ROWS][COLS];
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
JButton button = new JButton();
button.setPreferredSize(new java.awt.Dimension(BUTTON_WIDTH, BUTTON_HEIGHT));
button.setOpaque(true);
button.setBackground(Color.WHITE);
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JButton clickedButton = (JButton) e.getSource();
if (selectedButton == null) {
selectedButton = clickedButton;
selectedButton.setBackground(Color.LIGHT_GRAY);
} else if (selectedButton == clickedButton) {
selectedButton.setBackground(Color.WHITE);
selectedButton = null;
} else if (selectedButton.getText().equals(clickedButton.getText())) {
selectedButton.setBackground(Color.GREEN);
clickedButton.setBackground(Color.GREEN);
selectedButton.setEnabled(false);
clickedButton.setEnabled(false);
selectedButton = null;
score += 10;
movesLeft--;
checkEndGame();
} else {
selectedButton.setBackground(Color.WHITE);
selectedButton = clickedButton;
selectedButton.setBackground(Color.LIGHT_GRAY);
movesLeft--;
checkEndGame();
}
updateScoreLabel();
updateMovesLeftLabel();
}
});
mainPanel.add(button);
buttons[i][j] = button;
}
}
JPanel scorePanel = new JPanel(new BorderLayout());
scorePanel.add(createScoreLabel(), BorderLayout.WEST);
scorePanel.add(createMovesLeftLabel(), BorderLayout.EAST);
add(mainPanel, BorderLayout.CENTER);
add(scorePanel, BorderLayout.SOUTH);
pack();
setLocationRelativeTo(null);
setVisible(true);
movesLeft = ROWS * COLS / 2;
}
private void checkEndGame() {
if (movesLeft == 0) {
JOptionPane.showMessageDialog(this, "Game Over! Your score is " + score + ".");
System.exit(0);
}
}
private void updateScoreLabel() {
((JButton) getContentPane().getComponent(ROWS * COLS)).setText("Score: " + score);
}
private void updateMovesLeftLabel() {
((JButton) getContentPane().getComponent(ROWS * COLS + 1)).setText("Moves Left: " + movesLeft);
}
private JButton createScoreLabel() {
JButton scoreLabel = new JButton("Score: 0");
scoreLabel.setPreferredSize(new java.awt.Dimension(100, BUTTON_HEIGHT));
scoreLabel.setFocusable(false);
scoreLabel.setEnabled(false);
return scoreLabel;
}
private JButton createMovesLeftLabel() {
JButton movesLeftLabel = new JButton("Moves Left: " + movesLeft);
movesLeftLabel.setPreferredSize(new java.awt.Dimension(100, BUTTON_HEIGHT));
movesLeftLabel.setFocusable(false);
movesLeftLabel.setEnabled(false);
return movesLeftLabel;
}
public static void main(String[] args) {
new LetterLinkGame();
}
}
```
该游戏的基本思路是:将所有字母随机打乱并排列在按钮上,玩家需要选择两个相同的字母按钮并将它们消除,直到所有字母按钮都被消除为止。在游戏过程中,程序会记录玩家的分数和剩余步数,并在所有字母按钮都被消除时结束游戏。
相关推荐
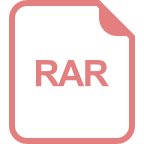
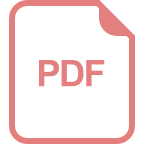
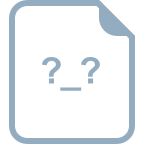














