我要实现一个功能,基于STM32F101C8T6芯片驱动断码式LCD屏,使用标准库开发,这个屏是东鑫达电子有限公司的DXDTN3320型号,有3个COM口和68个SEG口,帮我编写相关代码
时间: 2023-12-14 14:03:26 浏览: 50
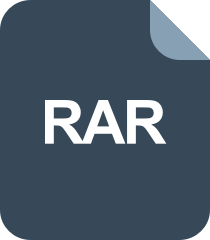
STM32F103C8t6(llc)控制0.96OLED显示屏,stm32f103c8t6引脚功能,C,C++

好的,以下是一个简单的示例代码,可以实现使用 STM32F101C8T6 芯片驱动断码式 LCD 屏,使用标准库开发,这个屏是东鑫达电子有限公司的 DXDTN3320 型号,有 3 个 COM 口和 68 个 SEG 口的功能:
```c
#include "stm32f10x.h" // 包含 STM32F10x 标准库头文件
#define SEGMENT_GPIO GPIOB // 定义 SEG 端口所在 GPIO
#define COM_GPIO GPIOC // 定义 COM 端口所在 GPIO
#define SEGMENT_PIN_LOW 0 // SEG 端口低位
#define SEGMENT_PIN_HIGH 1 // SEG 端口高位
#define COM_PIN_1 0 // COM1 端口
#define COM_PIN_2 1 // COM2 端口
#define COM_PIN_3 2 // COM3 端口
void LCD_Init(void)
{
// 初始化 SEG 端口为推挽输出
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = (1 << SEGMENT_PIN_LOW) | (1 << SEGMENT_PIN_HIGH);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(SEGMENT_GPIO, &GPIO_InitStructure);
// 初始化 COM 端口为推挽输出
GPIO_InitStructure.GPIO_Pin = (1 << COM_PIN_1) | (1 << COM_PIN_2) | (1 << COM_PIN_3);
GPIO_Init(COM_GPIO, &GPIO_InitStructure);
}
void LCD_Display(uint8_t number)
{
static const uint8_t SEGMENT_DATA[] = {
// 0~9 数字的 SEG 端口数据
0b00111111, // 0
0b00000110, // 1
0b01011011, // 2
0b01001111, // 3
0b01100110, // 4
0b01101101, // 5
0b01111101, // 6
0b00000111, // 7
0b01111111, // 8
0b01101111, // 9
};
// 获取当前数字对应的 SEG 端口数据
uint8_t segment_data = SEGMENT_DATA[number % 10];
// 输出 SEG 端口数据
GPIO_WriteBit(SEGMENT_GPIO, (1 << SEGMENT_PIN_LOW), ((segment_data & 0x01) == 0x01) ? Bit_SET : Bit_RESET);
GPIO_WriteBit(SEGMENT_GPIO, (1 << SEGMENT_PIN_HIGH), ((segment_data & 0x02) == 0x02) ? Bit_SET : Bit_RESET);
// 循环输出 COM 端口数据
GPIO_WriteBit(COM_GPIO, (1 << COM_PIN_1), Bit_RESET);
GPIO_WriteBit(COM_GPIO, (1 << COM_PIN_2), Bit_RESET);
GPIO_WriteBit(COM_GPIO, (1 << COM_PIN_3), Bit_RESET);
for (uint8_t i = 0; i < 3; i++) {
GPIO_WriteBit(COM_GPIO, (1 << i), Bit_SET);
for (volatile uint32_t j = 0; j < 100; j++); // 短暂延时,用于控制显示刷新速度
}
}
int main(void)
{
// 初始化系统时钟
SystemInit();
// 初始化 LCD 屏幕
LCD_Init();
// 循环显示数字 0~9
uint8_t number = 0;
while (1) {
LCD_Display(number);
number = (number + 1) % 10;
}
}
```
以上代码仅供参考,具体实现还需要根据实际硬件连接和需求进行调整。
阅读全文
相关推荐

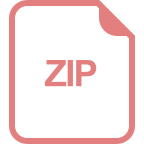
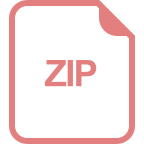
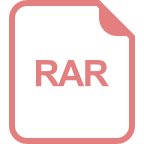
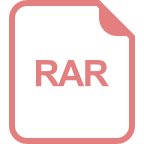
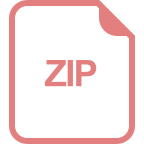
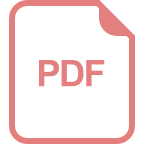
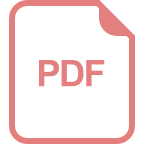
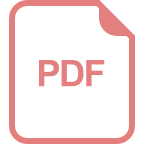
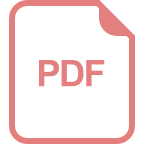
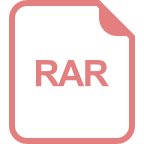
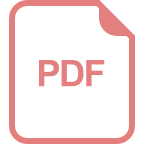
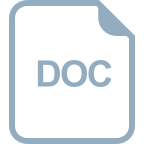
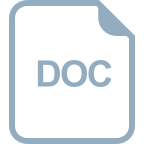
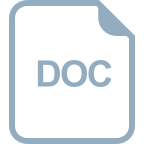