用c语言写出学院考勤管理系统
时间: 2023-11-11 08:05:38 浏览: 38
以下是一个简单的学院考勤管理系统C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STUDENTS 100
#define MAX_NAME_LENGTH 50
#define MAX_ID_LENGTH 20
struct student {
char name[MAX_NAME_LENGTH];
char id[MAX_ID_LENGTH];
int presence_count;
};
void add_student(struct student *students, int *student_count) {
if (*student_count >= MAX_STUDENTS) {
printf("The maximum number of students has been reached.\n");
return;
}
struct student new_student;
printf("Enter student name: ");
scanf("%s", new_student.name);
printf("Enter student ID: ");
scanf("%s", new_student.id);
new_student.presence_count = 0;
students[*student_count] = new_student;
(*student_count)++;
printf("Student added successfully.\n");
}
void mark_presence(struct student *students, int student_count) {
char id[MAX_ID_LENGTH];
printf("Enter student ID: ");
scanf("%s", id);
for (int i = 0; i < student_count; i++) {
if (strcmp(students[i].id, id) == 0) {
students[i].presence_count++;
printf("Presence marked for student %s.\n", students[i].name);
return;
}
}
printf("No student found with ID %s.\n", id);
}
void print_report(struct student *students, int student_count) {
printf("Student ID\tName\tPresence Count\n");
for (int i = 0; i < student_count; i++) {
printf("%s\t%s\t%d\n", students[i].id, students[i].name, students[i].presence_count);
}
}
int main() {
struct student students[MAX_STUDENTS];
int student_count = 0;
int choice;
while (1) {
printf("\n1. Add student\n");
printf("2. Mark presence\n");
printf("3. Print report\n");
printf("4. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_student(students, &student_count);
break;
case 2:
mark_presence(students, student_count);
break;
case 3:
print_report(students, student_count);
break;
case 4:
exit(0);
default:
printf("Invalid choice.\n");
}
}
return 0;
}
```
该程序实现了一个简单的学院考勤管理系统,可以添加学生、标记学生出席情况和打印出席报告。
相关推荐
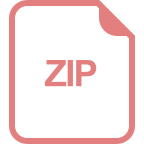
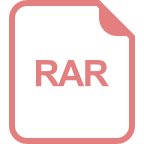
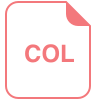
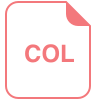
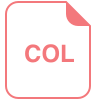
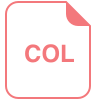
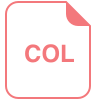









