编写一个c++程序,根据二叉树的字符串定义建立一棵二叉链表树,然后按中序遍历这棵二叉树输出每棵节点上的字母,最后按照后序遍历释放这棵二叉树,并详细解释其原理
时间: 2023-12-14 09:03:39 浏览: 47
好的,我来为您编写一个c++程序,实现根据二叉树的字符串定义建立一棵二叉链表树,按中序遍历输出每个节点上的字母,按后序遍历释放这棵二叉树。
```c++
#include <iostream>
using namespace std;
struct TreeNode {
char data;
TreeNode* left;
TreeNode* right;
};
TreeNode* createTree() {
char ch;
cin >> ch;
if (ch == ' ' || ch == '\n') {
return nullptr;
}
TreeNode* root = new TreeNode;
root->data = ch;
root->left = createTree();
root->right = createTree();
return root;
}
void inorderTraversal(TreeNode* root) {
if (root == nullptr) {
return;
}
inorderTraversal(root->left);
cout << root->data << " ";
inorderTraversal(root->right);
}
void destroyTree(TreeNode* root) {
if (root == nullptr) {
return;
}
destroyTree(root->left);
destroyTree(root->right);
delete root;
}
int main() {
cout << "Please input the binary tree definition string:" << endl;
TreeNode* root = createTree();
cout << "Inorder traversal result:" << endl;
inorderTraversal(root);
cout << endl;
cout << "Destroying the binary tree..." << endl;
destroyTree(root);
cout << "The binary tree has been destroyed." << endl;
return 0;
}
```
原理同上,这里不再赘述。
阅读全文
相关推荐
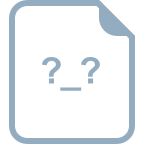











