利用Eigen库实现obb盒
时间: 2024-01-14 09:03:13 浏览: 436
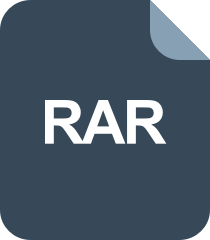
obb和obb树代码,使用EIGEN处理矩阵。
首先,需要理解obb盒的概念和计算方法。obb盒是一种用于包围物体的盒子,它的面朝物体的表面,可以用来快速计算物体的碰撞和运动。obb盒的计算方法通常是通过求解物体的协方差矩阵,然后求解矩阵的特征值和特征向量,进而计算obb盒的位置和方向。
在Eigen库中,可以使用Matrix类来表示矩阵,并使用SelfAdjointEigenSolver类来求解特征值和特征向量。下面是一个示例代码,用于计算obb盒的位置、方向和大小。
```c++
#include <Eigen/Dense>
#include <Eigen/Eigenvalues>
using namespace Eigen;
void computeOBB(const MatrixXf& points, Vector3f& position, Matrix3f& orientation, Vector3f& halfExtents)
{
int numPoints = points.cols();
// Compute the center of mass
Vector3f center = points.rowwise().mean();
// Compute the covariance matrix
Matrix3f cov = Matrix3f::Zero();
for (int i = 0; i < numPoints; i++)
{
Vector3f p = points.col(i) - center;
cov += p * p.transpose();
}
cov /= numPoints;
// Compute the eigenvectors and eigenvalues
SelfAdjointEigenSolver<Matrix3f> solver(cov);
Vector3f eigenvalues = solver.eigenvalues();
Matrix3f eigenvectors = solver.eigenvectors();
// Set the position to the center of mass
position = center;
// Set the orientation to the eigenvectors
orientation = eigenvectors;
// Set the half extents to the square roots of the eigenvalues
halfExtents = eigenvalues.array().sqrt();
}
```
在这个示例代码中,MatrixXf表示一个矩阵,其中每一列代表一个点的坐标。Vector3f和Matrix3f分别表示一个三维向量和一个三维矩阵。SelfAdjointEigenSolver类用于求解协方差矩阵的特征值和特征向量。
在函数的实现中,首先计算点云的中心点,然后计算协方差矩阵。接着,使用SelfAdjointEigenSolver类求解特征值和特征向量。最后,将中心点、特征向量和特征值的平方根设置为obb盒的位置、方向和大小。
需要注意的是,这个示例代码中没有考虑obb盒的旋转角度。如果需要计算obb盒的旋转角度,可以使用Quaternion类来表示四元数,并计算特征向量之间的旋转矩阵。
阅读全文
相关推荐
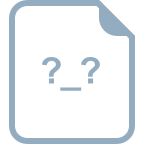
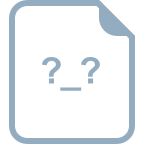




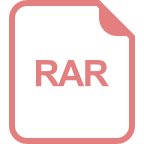
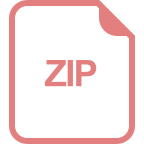
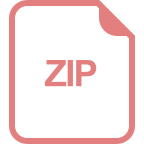
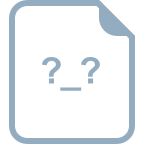
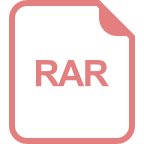






