本实验实现父子进程之间的管道通信。程序中由父进程创建一个匿名管道,并创建了2个子进程。两个子进程都从管道写端(入口)写入一个发给父进程的消息(字符串),父进程则从管道读端(出口)读取发来的两个消息。 三、实验主要步骤 (1)程序设计 下面是程序的部分代码,请完成该程序的设计。 /*test5_1.c:匿名管道*/ # include <stdio.h> # include <unistd.h> int main( ) { int i ,p1 ,p2 , pid , fd[2] ; char buf[80]; <创建一个匿名管道> while((p1=fork( ))==-1); if (p1==0) { /*子进程1代码*/ sprintf (buf,”Message of child_1”); /*在buf中写入消息文本*/ <向管道写入消息> printf (“Child_1 write an message to pipe!\n”); for( i=0; i<99999; i++); <终止> } else{ while((p2=fork())==-1); if (p2==0){ /*子进程2代码*/ sprintf (buf,”Message of child_2”); <向管道写入消息> printf (“Child_2 write an message to pipe!\n”); for( i=0; i<99999; i++); <终止> } else { /*父进程代码*/ <等待子进程终止> <从管道中先后读出两个消息,并输出相关提示信息> <关闭管道> printf(“OVER\n”); } } return 0; } 为什么前两行的顺序可颠倒,第3和第4行的顺序也可颠倒?帮我分析一下
时间: 2024-02-15 09:27:42 浏览: 75
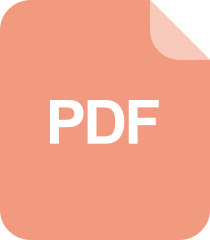
UNIX 操作系统基础实验 - 利用管道实现两个进程的通信.pdf
前两行的顺序(# include <stdio.h> 和 # include <unistd.h>)可以颠倒,因为它们都是头文件,不会相互影响,只要在程序中能正确引用它们的函数即可。第3和第4行的顺序也可以颠倒,因为创建匿名管道时需要一个int类型的数组来存储读写端的文件描述符,而数组的初始化是在第4行完成的,所以第4行的执行要在第3行之前。
阅读全文
相关推荐
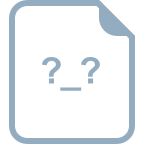
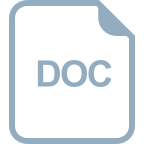
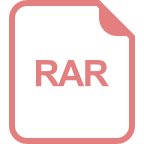
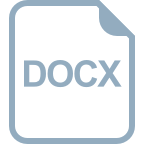
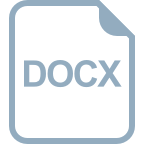
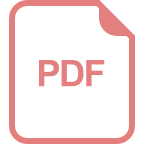
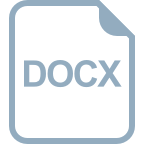
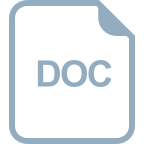
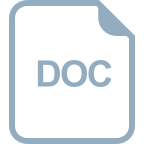
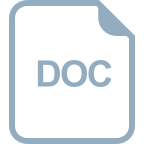
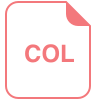
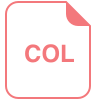
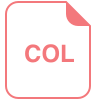
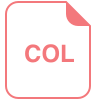
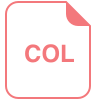
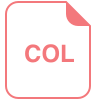

