上面的要求使用unet
时间: 2024-04-20 18:26:26 浏览: 7
如果你想使用U-Net模型来提取标注部分,可以借助深度学习库如TensorFlow或PyTorch来实现。下面是一个使用PyTorch的示例代码:
```python
import torch
import torch.nn as nn
import torchvision.transforms as transforms
from PIL import Image
# 定义U-Net模型
class UNet(nn.Module):
def __init__(self):
super(UNet, self).__init__()
# 定义U-Net的结构,包括编码器和解码器部分
def forward(self, x):
# 前向传播过程
# 加载预训练的U-Net模型
model = UNet()
model.load_state_dict(torch.load('path_to_pretrained_model.pth'))
model.eval()
# 定义图像转换
transform = transforms.Compose([
transforms.ToTensor(),
# 添加其他必要的图像预处理操作
])
def extract_annotation(image_path, annotation_coords, output_path):
# 读取原始图片
image = Image.open(image_path)
# 将图片转换为模型输入的张量
image_tensor = transform(image).unsqueeze(0)
# 使用U-Net模型进行预测
with torch.no_grad():
prediction = model(image_tensor)
# 提取标注部分
x, y, w, h = annotation_coords # 标注的左上角坐标和宽高
annotation = prediction[:, :, y:y+h, x:x+w]
# 将标注部分转换为PIL图像并保存
output_image = transforms.ToPILImage()(annotation.squeeze(0))
output_image.save(output_path)
# 示例用法
image_path = 'path_to_image.jpg' # 原始图片路径
annotation_coords = (100, 100, 200, 200) # 标注的左上角坐标和宽高
output_path = 'path_to_output_image.jpg' # 输出图片路径
extract_annotation(image_path, annotation_coords, output_path)
```
在这个示例中,我们首先定义了一个U-Net模型,并加载了预训练的权重。然后,我们通过`transforms.Compose`定义了图像转换的操作,以便将输入图像转换为模型可以处理的张量格式。在`extract_annotation`函数中,我们使用PIL库读取原始图像,并将其转换为模型输入的张量。然后,通过模型进行预测,并从预测结果中提取标注部分。最后,我们将提取的标注部分转换为PIL图像,并保存到指定的路径。
请注意,上述代码只是一个简单示例,你需要根据具体情况来定义U-Net模型的结构和训练方法,并根据需要进行适当的调整和修改。
相关推荐
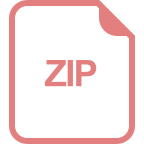
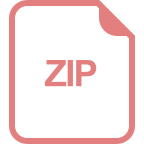














