index.vue界面中有一个表单,可以上传文件和选择单选框,然后点击提交按钮后要将文件和单选框的值传入django中,给出具体代码
时间: 2024-03-02 13:52:21 浏览: 80
下面是一个简单的示例代码,演示了如何在Vue中上传文件和选择单选框,并将数据传递给Django后端:
在Vue中的 index.vue 文件:
```html
<template>
<div>
<form @submit.prevent="submitForm">
<label>
<input type="file" ref="fileInput" @change="handleFileChange">
</label>
<label>
<input type="radio" v-model="radioValue" value="option1"> Option 1
</label>
<label>
<input type="radio" v-model="radioValue" value="option2"> Option 2
</label>
<button type="submit">Submit</button>
</form>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
radioValue: '',
file: null
};
},
methods: {
handleFileChange(event) {
this.file = event.target.files[0];
},
submitForm() {
let formData = new FormData();
formData.append('file', this.file);
formData.append('radioValue', this.radioValue);
axios.post('/api/submit-form/', formData, {
headers: {
'Content-Type': 'multipart/form-data'
}
}).then(response => {
console.log(response.data);
}).catch(error => {
console.log(error);
});
}
}
};
</script>
```
在Django中的 views.py 文件:
```python
from django.http import JsonResponse
from django.views.decorators.csrf import csrf_exempt
@csrf_exempt
def submit_form(request):
if request.method == 'POST':
file = request.FILES.get('file')
radio_value = request.POST.get('radioValue')
# 处理上传的文件和单选框的值
# ...
return JsonResponse({'success': True})
else:
return JsonResponse({'error': 'Method Not Allowed'}, status=405)
```
在上面的示例代码中,我们通过`FormData`对象将文件和单选框的值一起提交到Django后端,然后在Django中的视图函数中使用`request.FILES`和`request.POST`来获取上传的文件和单选框的值。你可以根据自己的需求来调整这些代码。
阅读全文
相关推荐
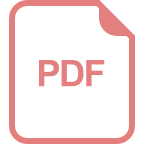
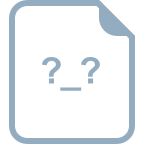
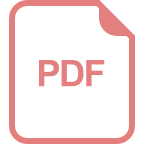


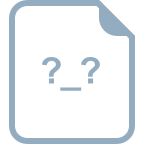
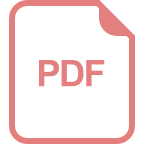
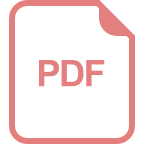
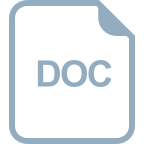
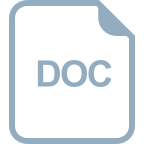
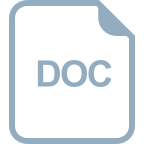
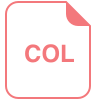





