springBoot集成mybatis
时间: 2023-05-25 10:06:52 浏览: 107
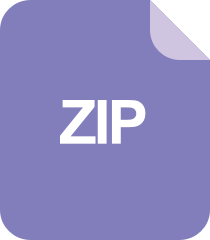
SpringBoot 集成 Mybatis
Spring Boot提供了自动集成MyBatis的便捷方式,主要涉及以下步骤:
1. 添加依赖:在pom.xml中添加MyBatis和MyBatis-Spring的依赖。
```
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.7</version>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.4</version>
</dependency>
```
2. 配置数据源:在application.properties或application.yml中配置数据源。
```
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/db_name?useUnicode=true&characterEncoding=UTF-8&serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=root
```
3. 配置MyBatis:通过添加@MapperScan注解或在MyBatis配置文件中指定映射器的位置,让Spring Boot自动扫描和注册MyBatis映射器。
```
@MapperScan(basePackages = "com.example.demo.mapper")
```
或者在mybatis-config.xml中添加:
```
<mappers>
<mapper class="com.example.demo.mapper.UserMapper"/>
<mapper class="com.example.demo.mapper.OrderMapper"/>
</mappers>
```
4. 编写对应的Mapper接口和XML文件:根据需要编写对应的Mapper接口和XML文件。
```
public interface UserMapper {
User getUserById(Long id);
}
<select id="getUserById" parameterType="java.lang.Long" resultType="com.example.demo.model.User">
select * from user where id = #{id}
</select>
```
5. 在Service类中注入Mapper:通过@Autowired注解将MyBatis映射器注入到Service类中,以便使用Mapper中的方法。
```
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public User getUserById(Long id) {
return userMapper.getUserById(id);
}
}
```
6. 启动应用程序:使用Spring Boot的自动配置和启动机制启动应用程序。
以上是基本的Spring Boot集成MyBatis的步骤,当然还涉及到事务、分页、动态SQL等更多的内容。总体而言,使用Spring Boot集成MyBatis可以非常方便地开发持久层部分,提高开发效率。
阅读全文
相关推荐
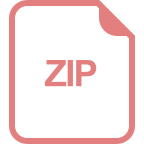
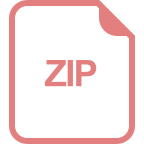
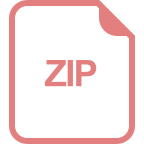
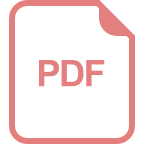
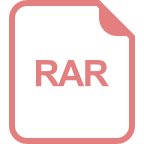
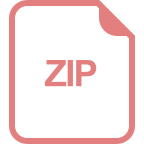
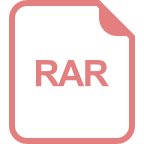
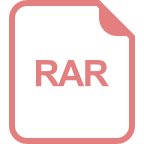
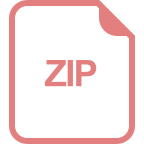
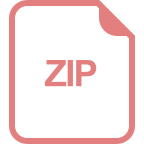
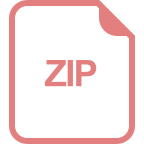



