c++ powerpoint 文本框插入公式 代码
时间: 2023-08-24 11:29:57 浏览: 63
要在 C++ PowerPoint 中使用 VBA 代码插入数学公式,请按照以下步骤操作:
1. 打开 PowerPoint 并进入 VBA 编辑器。您可以按 ALT + F11 快捷键打开 VBA 编辑器窗口。
2. 在 VBA 编辑器中,选择“插入”菜单,然后选择“模块”选项。
3. 在新模块中输入以下 VBA 代码:
```
Sub InsertEquation()
Dim oShp As Shape
Set oShp = ActiveWindow.Selection.ShapeRange(1)
oShp.TextFrame.TextRange.InsertFormula "Insert your equation here"
oShp.TextFrame2.AutoSize = msoAutoSizeTextToFitShape
End Sub
```
4. 在代码中,替换 "Insert your equation here" 为你要插入的数学公式。
5. 保存您的 VBA 模块,并关闭 VBA 编辑器。
6. 在 PowerPoint 中打开您的演示文稿,并在要插入公式的幻灯片上创建一个文本框。
7. 选中文本框,并按下 ALT + F8 快捷键打开“宏”对话框。
8. 选择您刚刚创建的宏,并单击“运行”按钮。
9. 现在,您的数学公式将插入到您创建的文本框中。
希望这些步骤能够帮助您在 C++ PowerPoint 中使用 VBA 代码成功插入数学公式。
相关问题
c++ powerpoint 文本框插入公式
要在 C++ Powerpoint 中插入公式,可以使用 Microsoft Office 提供的 COM 接口来实现。具体步骤如下:
1. 首先需要在 C++ 代码中引入 Office 相关的头文件和库文件,比如:
```c++
#include <Windows.h>
#include <ole2.h>
#include <comutil.h>
#include <comdef.h>
#include <atlbase.h>
#pragma comment(lib, "ole32.lib")
#pragma comment(lib, "comsupp.lib")
```
2. 接着需要创建一个 PowerPoint 应用程序对象,代码如下:
```c++
HRESULT hr;
CLSID clsid;
hr = CLSIDFromProgID(L"PowerPoint.Application", &clsid);
if (FAILED(hr)) {
// 创建对象失败
return -1;
}
CComPtr<IDispatch> pAppDisp;
hr = CoCreateInstance(clsid, NULL, CLSCTX_LOCAL_SERVER, IID_PPV_ARGS(&pAppDisp));
if (FAILED(hr)) {
// 创建对象失败
return -1;
}
CComQIPtr<_Application> pPPTApp(pAppDisp);
if (pPPTApp == nullptr) {
// 转换对象类型失败
return -1;
}
// 设置 PowerPoint 应用程序的 Visible 属性为 True,以便在界面上显示
pPPTApp->put_Visible(VARIANT_TRUE);
```
3. 创建一个新的演示文稿,并打开该演示文稿,代码如下:
```c++
CComPtr<_Presentation> pPPTPresentation;
hr = pPPTApp->Presentations->Add(VARIANT_FALSE, &pPPTPresentation);
if (FAILED(hr)) {
// 创建演示文稿失败
return -1;
}
// 打开演示文稿
hr = pPPTPresentation->Open(L"path/to/your/presentation.pptx", VARIANT_FALSE, VARIANT_FALSE, VARIANT_FALSE);
if (FAILED(hr)) {
// 打开演示文稿失败
return -1;
}
```
4. 在演示文稿的某个幻灯片中添加一个文本框,代码如下:
```c++
// 获取演示文稿中的第一个幻灯片
CComPtr<_Slide> pSlide;
hr = pPPTPresentation->Slides->Item(1, &pSlide);
if (FAILED(hr)) {
// 获取幻灯片失败
return -1;
}
// 在幻灯片中添加一个文本框
CComPtr<Shapes> pShapes;
hr = pSlide->get_Shapes(&pShapes);
if (FAILED(hr)) {
// 获取幻灯片上的 Shapes 对象失败
return -1;
}
CComPtr<Shape> pTextBox;
hr = pShapes->AddTextbox(msoTextOrientationHorizontal, 100, 100, 400, 200, &pTextBox);
if (FAILED(hr)) {
// 添加文本框失败
return -1;
}
```
5. 在文本框中插入公式,代码如下:
```c++
// 获取文本框的 TextFrame 对象
CComPtr<TextFrame> pTextFrame;
hr = pTextBox->get_TextFrame(&pTextFrame);
if (FAILED(hr)) {
// 获取 TextFrame 对象失败
return -1;
}
// 在 TextFrame 中添加一个段落
CComPtr<TextRange> pTextRange;
hr = pTextFrame->get_TextRange(&pTextRange);
if (FAILED(hr)) {
// 获取 TextRange 对象失败
return -1;
}
CComPtr<TextRange> pParagraphRange;
hr = pTextRange->InsertAfter(L"", &pParagraphRange);
if (FAILED(hr)) {
// 添加段落失败
return -1;
}
// 在段落中插入公式
CComPtr<TextRange> pFormulaRange;
hr = pParagraphRange->InsertFormula(L"公式", VARIANT_TRUE, &pFormulaRange);
if (FAILED(hr)) {
// 插入公式失败
return -1;
}
```
其中,公式可以是 LaTeX 语法格式的字符串,比如 `$\frac{a}{b}$`。
6. 最后,释放创建的对象和资源,代码如下:
```c++
// 释放对象
pFormulaRange.Release();
pParagraphRange.Release();
pTextRange.Release();
pTextFrame.Release();
pTextBox.Release();
pShapes.Release();
pSlide.Release();
pPPTPresentation.Release();
pPPTApp.Release();
// 释放 COM 库
CoUninitialize();
```
需要注意的是,在使用 COM 接口时,需要手动管理对象的生命周期和释放资源,否则容易导致内存泄漏和程序崩溃等问题。
c++ powerpoint 文本框插入公式 例子
以下是一个使用 C++ 和 VBA 代码将数学公式插入 PowerPoint 文本框的示例:
```
#include <windows.h>
#include <ole2.h>
#include <atlbase.h>
#include <comutil.h>
#include <cstdio>
#pragma comment(lib, "ole32.lib")
#pragma comment(lib, "comsuppw.lib")
int main()
{
HRESULT hr = CoInitialize(NULL);
if (FAILED(hr))
{
printf("Failed to initialize COM library\n");
return 1;
}
hr = CoCreateInstance(CLSID_PowerPointApplication, NULL, CLSCTX_LOCAL_SERVER, IID_IDispatch, (void**)&app);
if (FAILED(hr))
{
printf("Failed to create PowerPoint application instance\n");
CoUninitialize();
return 1;
}
// 打开一个演示文稿
IDispatch* pres = NULL;
_variant_t vtName("C:\\example.pptx");
hr = app->Presentations->Open(vtName, VARIANT_FALSE, VARIANT_FALSE, VARIANT_FALSE, &pres);
if (FAILED(hr))
{
printf("Failed to open presentation\n");
app->Release();
CoUninitialize();
return 1;
}
// 在幻灯片上插入一个文本框
IDispatch* slide = NULL;
hr = pres->Slides->Item(1, &slide);
if (FAILED(hr))
{
printf("Failed to get slide\n");
pres->Close();
app->Release();
CoUninitialize();
return 1;
}
IDispatch* txtBox = NULL;
hr = slide->Shapes->AddTextbox(msoTextOrientationHorizontal, 100, 100, 400, 100, &txtBox);
if (FAILED(hr))
{
printf("Failed to add text box\n");
slide->Release();
pres->Close();
app->Release();
CoUninitialize();
return 1;
}
// 将数学公式插入文本框
_bstr_t formula(L"y = mx + b"); // 更改此处的公式
IDispatch* txtRange = NULL;
hr = txtBox->GetItem(_variant_t(2), &txtRange);
if (FAILED(hr))
{
printf("Failed to get text range\n");
txtBox->Release();
slide->Release();
pres->Close();
app->Release();
CoUninitialize();
return 1;
}
hr = txtRange->CallMethod(DISPID_VALUE, NULL, 1, &formula);
if (FAILED(hr))
{
printf("Failed to insert formula\n");
txtRange->Release();
txtBox->Release();
slide->Release();
pres->Close();
app->Release();
CoUninitialize();
return 1;
}
// 保存并关闭演示文稿
pres->Save();
pres->Close();
// 释放 COM 对象
txtRange->Release();
txtBox->Release();
slide->Release();
pres->Release();
app->Release();
CoUninitialize();
return 0;
}
```
这是一个使用 C++ 和 VBA 代码向 PowerPoint 文本框插入数学公式的简单示例。它使用 PowerPoint 对象模型和 VBA 代码来打开演示文稿、插入文本框、插入数学公式并保存演示文稿。请注意,您需要将代码中的公式更改为您要插入的实际公式。
相关推荐
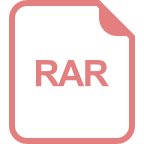
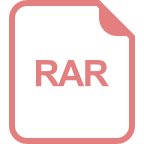
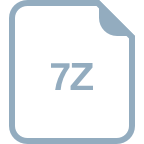












