C语言统计并输出两个字符串共有多少相同的字母以及出现次数
时间: 2024-10-14 14:08:29 浏览: 14
在C语言中,你可以通过以下几个步骤来统计并输出两个字符串中共有的字母及其出现次数:
1. **初始化**:首先,定义两个字符数组存储输入的字符串,并分配足够的空间来容纳可能存在的所有字符。
```c
#include <stdio.h>
#include <string.h>
#define MAX_CHAR 100 // 预设最大字符数
char str1[MAX_CHAR], str2[MAX_CHAR];
```
2. **获取输入**:从用户那里获取两个字符串。
```c
scanf("%s%s", str1, str2);
```
3. **创建哈希表**:可以使用一个关联数组(如`int count[26]`,假设我们只考虑小写字母,ASCII码范围内的前26个字符)来记录每个字符的出现次数。初始化所有的计数值为0。
```c
int count[26] = {0};
```
4. **遍历字符串**:遍历第一个字符串,检查每个字符是否也在第二个字符串中,并更新计数器。
```c
for (int i = 0; str1[i] != '\0'; i++) {
char c = tolower(str1[i]); // 转换为小写便于比较
if (str2[i] != '\0' && c == tolower(str2[i])) {
count[c - 'a']++; // 更新对应的计数
}
}
```
5. **统计结果**:遍历完第一个字符串后,遍历哈希表并输出共同的字符及其出现次数。
```c
printf("共有的字母及出现次数:\n");
for (int i = 0; i < 26; i++) {
if (count[i] > 0) {
printf("'%c': %d\n", 'a' + i, count[i]);
}
}
```
6. **完整代码示例**:
```c
#include <stdio.h>
#include <ctype.h>
#define MAX_CHAR 100
// ...
void countCommonChars(char* str1, char* str2) {
int count[26] = {0};
for (int i = 0; str1[i] != '\0'; i++) {
char c = tolower(str1[i]);
if (str2[i] != '\0' && c == tolower(str2[i])) {
count[c - 'a']++;
}
}
printf("共有的字母及出现次数:\n");
for (int i = 0; i < 26; i++) {
if (count[i] > 0) {
printf("'%c': %d\n", 'a' + i, count[i]);
}
}
}
int main() {
scanf("%s%s", str1, str2);
countCommonChars(str1, str2);
return 0;
}
```
阅读全文
相关推荐
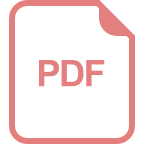
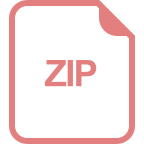
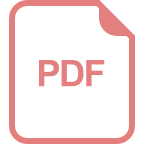















