Python 图中画双向箭头
时间: 2024-08-03 13:01:11 浏览: 164
在Python中,如果你想要在图表上绘制双向箭头,可以使用matplotlib库,特别是`pyplot`模块中的`annotate`函数。这个函数允许你在图像上添加文本注释,包括箭头。下面是一个简单的例子:
```python
import matplotlib.pyplot as plt
import numpy as np
# 创建数据
x = np.linspace(0, 10, 100)
y = x ** 2
# 绘制线图
plt.plot(x, y)
# 定义箭头的位置(x轴坐标,y轴坐标)
xytext = (5, 8) # 文本的位置
arrowprops = dict(facecolor='black', shrink=0.05) # 箭头属性
# 绘制双向箭头
plt.annotate('', xy=(7, 49), xycoords='data', # 起始点
xytext=xytext, textcoords='offset points', # 文本位置
arrowprops=arrowprops) # 添加箭头
plt.xlabel('X轴')
plt.ylabel('Y轴')
# 显示图形
plt.show()
```
在这个例子中,我们首先创建了一个简单的线图,然后在X轴坐标7处和对应的Y值49处绘制了一个双向箭头,从起点指向文本的位置。`arrowprops`参数用于设置箭头的颜色和缩放。
相关问题
python图上绘制双箭头,并使注释文本在两个箭头中间
要在 Python 图上绘制双箭头,并使注释文本在两个箭头中间,可以使用 `annotate()` 函数,并设置 `arrowstyle` 参数为 `'fancy, head_length=0.8, head_width=0.6, tail_width=0.4'`,这将创建一个双向箭头。在 `annotate()` 函数中设置 `xy` 参数为箭头的终点位置,设置 `xytext` 参数为注释文本的位置,并设置 `ha` 参数为 `'center'`,表示水平居中对齐。
以下是一个示例代码:
``` python
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
plt.plot(x, y)
plt.annotate('max value', xy=(5, 10), xytext=(3, 7),
arrowprops=dict(facecolor='black', arrowstyle='fancy, head_length=0.8, head_width=0.6, tail_width=0.4',
lw=1),
ha='center', fontsize=12)
plt.show()
```
这段代码将在图形中添加一个从点 (5, 10) 到点 (3, 7) 的双向箭头,并在箭头的中间添加了一段文本 "max value"。箭头的颜色为黑色,箭头样式为 fancy,箭头的头部长度为 0.8,头部宽度为 0.6,尾部宽度为 0.4,线宽为 1,注释文本的字体大小为 12,水平对齐方式为居中。
如果你想要将注释文本放置在双箭头的中间,可以设置 `xytext` 参数为一个元组,分别代表注释文本相对于箭头终点的 x 和 y 偏移量。在本例中,我们将 `xytext` 参数设置为 `(4, 8)`,表示注释文本相对箭头终点向左平移一些,向上平移一些。
根据a和b的值输出由*组成的双向箭头形状。 其中a是正奇数,代表图案有几行;b是正整数,代表双向箭头中间的横线有多长。
以下是根据a和b的值输出由*组成的双向箭头形状的示例代码:
```python
a = 5
b = 3
# 输出上半部分的双向箭头
for i in range(1, a+1):
if i <= (a+1)//2:
print('*' * (i+b))
else:
print('*' * (a+1-i+b))
# 输出下半部分的双向箭头
for i in range(a-1, 0, -1):
if i <= (a+1)//2:
print('*' * (i+b))
else:
print('*' * (a+1-i+b))
```
输出结果为:
```
*****
******
*******
******
*****
****
***
**
*
**
***
****
*****
******
*******
******
*****
```
阅读全文
相关推荐
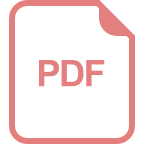
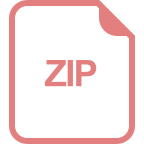
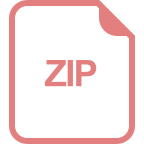
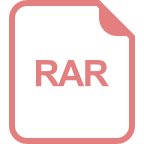
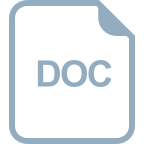
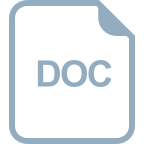
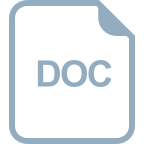
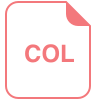
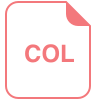
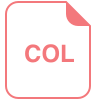
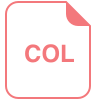
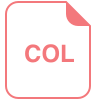
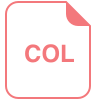
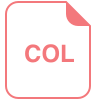


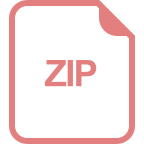