springboot配置aop
时间: 2023-10-14 19:56:11 浏览: 53
在Spring Boot中配置AOP(面向切面编程),可以通过以下步骤进行:
1. 首先,在你的Spring Boot项目中添加所需的依赖。在`pom.xml`文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
```
2. 创建一个切面类,用于定义你的切面逻辑。切面类需要使用`@Aspect`注解进行标记,并且通常需要在类中定义多个切点和通知。例如:
```java
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.demo.service.*.*(..))")
public void beforeAdvice(JoinPoint joinPoint) {
System.out.println("Before method: " + joinPoint.getSignature());
}
@After("execution(* com.example.demo.service.*.*(..))")
public void afterAdvice(JoinPoint joinPoint) {
System.out.println("After method: " + joinPoint.getSignature());
}
}
```
上述切面类中,`@Before`注解表示在目标方法执行之前执行,`@After`注解表示在目标方法执行之后执行。`execution(* com.example.demo.service.*.*(..))`表示匹配`com.example.demo.service`包下的所有类的所有方法。
3. 在Spring Boot应用程序的主类上添加`@EnableAspectJAutoProxy`注解,启用AOP代理。例如:
```java
@SpringBootApplication
@EnableAspectJAutoProxy
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
4. 运行你的Spring Boot应用程序,并观察控制台输出。你应该能够看到在匹配的方法执行前后打印的日志信息。
这就是在Spring Boot中配置AOP的基本步骤。你可以根据需要定义更多的切点和通知,以实现更复杂的切面逻辑。
相关推荐
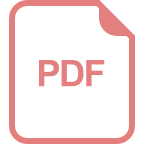
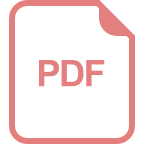
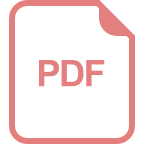














