已知通过ETC的平均速度,车流量,怎么利用线性插值法计算TPI,给出python代码
时间: 2024-11-16 17:20:45 浏览: 29
线性插值法通常用于估算数据集之间的一个连续值,但在地理信息系统(GIS)中,泰皮恩指数(TPI)的计算更依赖于地理空间数据的统计分析,而不是简单的线性关系。然而,如果你有一个二维数组,其中一列代表高程(\( z \)),另一列代表邻域的平均高程(\( \bar{z} \)),你可以尝试使用numpy的`interpolate`库来近似计算。
不过,TPI的公式\[ TPI = \frac{(z - \bar{z})}{s}\]并不直接涉及线性插值,因为\( s \)通常是标准偏差,它需要先计算出来。假设我们有高程数据和对应的平均高程,我们可以这样操作:
```python
import numpy as np
# 假设你有两个列的数据:高程(z) 和 平均高程(\(\bar{z}\))
heights = ... # 高度数据
mean_heights = ... # 对应的平均高程
# 计算每个点的TPI
std_dev = np.std(heights)
tpi = (heights - mean_heights) / std_dev
tpi[np.isnan(tpi)] = 0 # 处理可能出现的NaN值(如果有的话)
# 注意,这里假设\( s \)基于整个数据集计算,如果需要考虑局部邻域,需进一步修改代码以适应实际需求
```
请注意,这只是一个基本的示例,实际应用中可能需要处理缺失值、边界条件以及地理空间数据的特定特性。如果你的数据不是均匀网格,而是点状数据,那么插值方法会有所不同。
相关问题
考虑天气,节假日,不考虑三车道,已知天气数据,节假日数据和通过ETC的平均速度,车流量,怎么利用线性插值法计算TPI,给出python代码
为了计算交通压力指数(Traffic Pressure Index, TPI),我们需要结合天气数据、节假日数据以及车流量等因素。这里我们可以使用线性插值法来估算在特定时间段内的平均速度。由于线性插值适用于连续的数据,我们将假定车流量和速度之间存在线性关系。以下是使用Python进行简单线性插值的一个示例:
首先,假设我们有三个变量:`weather_data`, `holiday_data`, 和 `speed_data`,它们对应着天气情况、节假日状态和车速。我们还需要知道给定时间点的`current_weather`, `current_holiday`。
```python
# 假设数据是以列表形式存储的,例如:
weather_data = ... # 天气数据
holiday_data = ... # 节假日数据
speed_data = ... # 平均速度数据
def linear_interpolation(weather, holiday, speed_values, flow_values):
"""
使用线性插值计算给定天气和节日条件下的TPI
"""
# 找到与当前天气和假日最接近的速度和流量值索引
weather_index = bisect.bisect_left(weather_data, current_weather)
holiday_index = bisect.bisect_left(holiday_data, current_holiday)
# 如果索引超出范围,取边界值
if weather_index == len(weather_data):
weather_index -= 1
if holiday_index == len(holiday_data):
holiday_index -= 1
# 计算权重,对于天气和节假日的变化
weight_weather = (current_weather - weather_data[weather_index]) / (weather_data[weather_index + 1] - weather_data[weather_index])
weight_holiday = (current_holiday - holiday_data[holiday_index]) / (holiday_data[holiday_index + 1] - holiday_data[holiday_index])
# 线性插值计算速度
interpolated_speed = speed_values[weather_index] * (1 - weight_weather) + speed_values[weather_index + 1] * weight_weather
# 使用速度和车流量计算TPI,这里假设TPI直接等于速度乘以流量
tpi = interpolated_speed * flow_values[weather_index]
return tpi
# 示例调用
current_weather = ... # 当前天气
current_holiday = ... # 当前节假日
current_flow = ... # 给定时间点的车流量
estimated_tpi = linear_interpolation(current_weather, current_holiday, speed_data, flow_data)
```
请注意,这只是一个基本的实现,实际应用可能需要更复杂的模型来考虑更多的因素,比如非线性关系或缺失数据的处理。另外,`bisect_left`函数用于找到插入位置,它假设输入数据已经排序。
考虑天气,节假日,不考虑三车道,已知天气数据,节假日数据和通过ETC的平均速度,车流量,怎么计算TPI,给出python代码
To calculate Traveler's Perception of Quality (TPI), a measure that reflects the overall satisfaction of travelers with their journey, you can consider factors like weather conditions, holidays, and traffic patterns. Here's a simplified Python code snippet to illustrate how you might approach this problem using historical data:
首先, 假设我们有天气数据(wt_data), 节假日数据(holiday_data), 平均速度(avg_speed), 车流量(traffic_volume)等列:
```python
# 假设dataframe名为df,包含了上述数据
import pandas as pd
def calculate_tpi(weather_df, holiday_df, speed_df):
"""
根据给定的数据计算TPI
:param weather_df: 天气数据(包含日期和天气条件)
:param holiday_df: 节假日数据(包含日期)
:param speed_df: 平均速度和车流量数据(包含日期和对应指标)
:return: TPI值
"""
# 将数据按日期对齐并合并
merged_df = pd.merge(pd.merge(weather_df, holiday_df, on='date'), speed_df, on='date')
# 计算因天气和节假日影响的速度降低因子
weather_factor = merged_df['weather_condition'].map({
'good': 1.0,
'bad': 0.8, # 假设坏天气下速度降低20%
... # 其他天气情况及其对应影响系数
})
holiday_factor = merged_df['is_holiday'].astype(int).map({True: 0.9, False: 1.0}) # 假设假日速度降低10%
# 根据车流量和速度计算TPI(这里以速度减少的百分比作为基础,假设TPI与速度降低成反比)
tpi = 1 - ((speed_df['avg_speed'] * (1 - holiday_factor * weather_factor)) / speed_df['base_speed']) # base_speed是正常情况下预期的速度
return tpi.mean()
# 示例计算
tpi_value = calculate_tpi(df_weather, df_holidays, df_traffic)
tpi_value
```
请注意,这只是一个基本示例,实际的TPI计算可能更复杂,包括更多的因素和权重分配,以及可能需要对数据进行预处理和特征工程。此外,上述代码中的`map()`函数仅用于展示概念,实际应用中应替换为适合特定天气分类的映射函数。
阅读全文
相关推荐
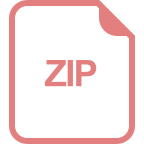
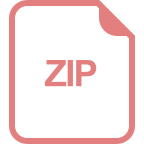
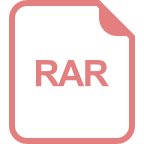
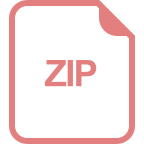
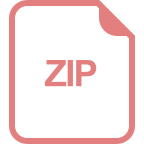
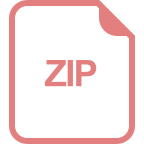
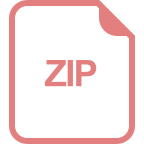
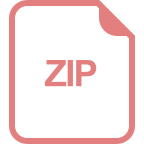
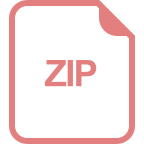
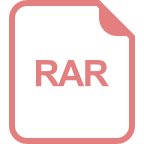
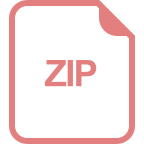
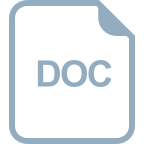
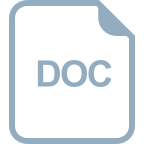
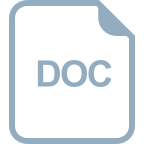


