用C代码编写差分链表的插入模块
时间: 2024-09-09 07:14:39 浏览: 56
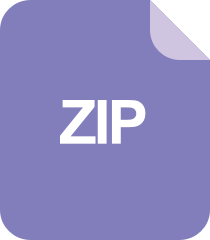
C语言编写的模拟歌手管理与打分系统.zip
差分链表是一种数据结构,用于高效地对数组或列表中的元素进行多次增减操作。在差分链表中,链表的每个节点代表数组中两个相邻元素的差值,而不是实际的元素值。这样,当我们需要在数组中插入或删除多个连续元素时,只需更新差分链表中的几个节点,而不需要移动整个数组中的元素。
下面是一个用C语言编写的差分链表插入模块的简单示例。这个示例假设链表中的节点包含一个`int`类型的差值`val`和一个指向下一个节点的指针`next`。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义差分链表的节点结构体
typedef struct DiffNode {
int val;
struct DiffNode *next;
} DiffNode;
// 创建一个新节点,初始差值为0
DiffNode* createNode() {
DiffNode* newNode = (DiffNode*)malloc(sizeof(DiffNode));
if (!newNode) {
printf("内存分配失败\n");
return NULL;
}
newNode->val = 0;
newNode->next = NULL;
return newNode;
}
// 在差分链表中插入一个新节点,并更新链表
// 参数:head指向链表头节点,position是新节点要插入的位置(从0开始计数),value是新节点的值
void insertAt(DiffNode* head, int position, int value) {
if (position < 0) {
printf("位置不能为负\n");
return;
}
DiffNode* newNode = createNode();
newNode->val = value;
if (position == 0) {
// 插入在链表的开头
newNode->next = head;
head = newNode;
} else {
// 在链表中间或末尾插入
DiffNode* current = head;
for (int i = 0; current != NULL && i < position - 1; i++) {
current = current->next;
}
if (current == NULL) {
printf("位置超出链表长度\n");
free(newNode);
return;
}
newNode->next = current->next;
current->next = newNode;
}
}
// 打印差分链表,用于调试
void printDiffList(DiffNode* head) {
DiffNode* current = head;
while (current != NULL) {
printf("%d ", current->val);
current = current->next;
}
printf("\n");
}
int main() {
// 创建一个空的差分链表
DiffNode* head = createNode();
// 插入操作示例
insertAt(head, 0, 1); // 在位置0插入差值1
insertAt(head, 1, -1); // 在位置1插入差值-1
insertAt(head, 2, 3); // 在位置2插入差值3
// 打印插入后的差分链表
printDiffList(head);
// 清理链表内存
DiffNode* current = head;
while (current != NULL) {
DiffNode* temp = current;
current = current->next;
free(temp);
}
return 0;
}
```
这个模块提供了创建新节点、在指定位置插入节点以及打印链表的功能。`insertAt`函数负责在链表的指定位置插入一个新节点,并更新前一个节点和新节点的值,以保持差分链表的特性。`printDiffList`函数用于打印整个差分链表,以便于调试。
阅读全文
相关推荐
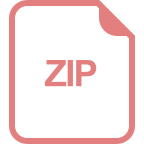
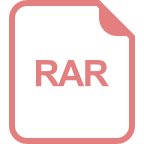

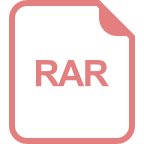
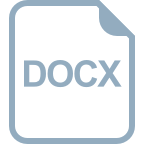
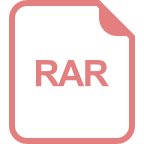
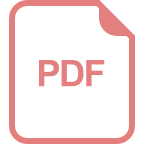
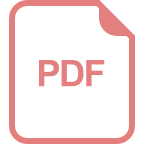
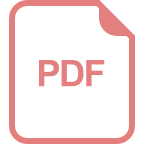
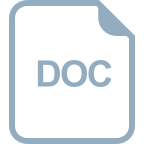
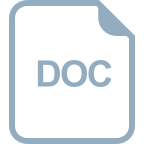
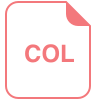
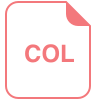
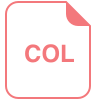
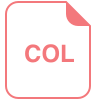

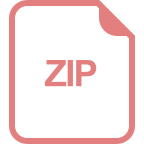