基于qt c++实现的主程序,如何实现将核心算法放入python运算,并将运算结果回传给主程序,给出具体完整的代码实际
时间: 2024-10-10 12:08:56 浏览: 20
在Qt C++中,如果你想要利用Python进行复杂计算并将结果返回到C++,可以使用PythonQt库来建立两者之间的桥梁。PythonQt允许你在C++应用程序中嵌入Python解释器并进行交互。首先,你需要安装PythonQt库(pip install pythonqt),然后按照以下步骤操作:
1. 首先,在C++中设置Python环境和初始化PythonQt模块:
```cpp
#include <PythonQt.h>
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
PythonQt::initPython(argc, argv);
// 创建Python interpreter对象
PythonInterpreter* interp = new PythonInterpreter();
if (!interp->initialize())
return -1;
// 确保Python环境准备好
interp->exec("import sys; print(sys.version)");
}
```
2. 使用PythonQt创建一个Python对象,包含你的核心算法函数:
```cpp
QObject* pyCoreModule = new QObject();
PyCallablePtr coreFunction = interp->newMethodCall("your_module", "core_algorithm"); // 替换为你的Python模块名和函数名
// 当需要调用Python算法时
QObject::connect(pyCoreModule, SIGNAL(resultReady(PyQObject*, PyObject*)), this, SLOT(onResultReady(PyQObject*, PyObject*)),
// 传递参数给Python函数(假设参数是一个列表)
Qt::QueuedConnection, Q_ARG(QList<int>, yourArguments));
```
3. 定义槽函数onResultReady()来接收Python函数的结果:
```cpp
void MainWindow::onResultReady(PyQObject* sender, PyObject* result)
{
// 将Python对象转换为C++类型
QVariant variantResult = result;
int computedValue = variantResult.toInt(); // 如果结果是整数
// 打印或处理计算结果
qDebug() << "Computed value: " << computedValue;
// 清理Python资源
delete sender;
delete interp;
}
```
4. 最后,启动Qt应用:
```cpp
if (app.exec() != 0)
{
delete interp;
delete pyCoreModule;
return 0;
}
return 0;
```
注意:这只是一个基本的示例,实际应用中可能还需要处理错误、异常以及更复杂的通信机制。同时,确保Python库在运行时可用,并且在部署时将其作为依赖一同打包。
阅读全文
相关推荐
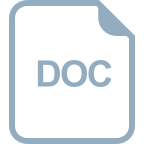
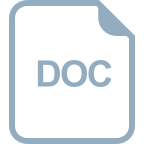
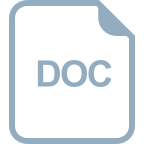
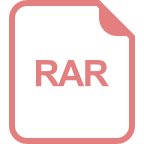
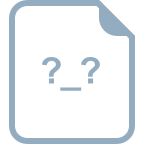
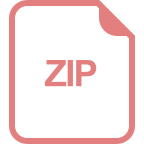
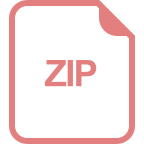
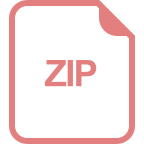
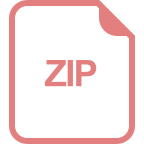
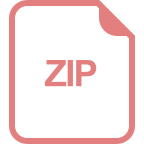
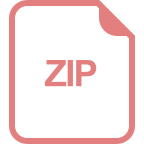
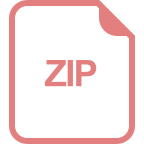
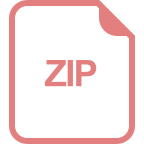
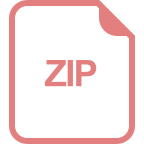
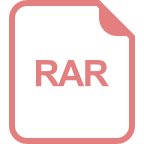
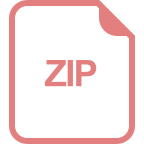
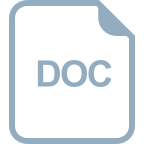