用C语言写程序:有100种商品的数据记录,每个记录包括“商品编号”、“商品名”、“单价”和“数量”,请用结构体数组实现每种商品总价的计算(商品总价=单价*数量)
时间: 2023-10-10 08:07:46 浏览: 29
好的,下面是用 C 语言实现这个程序的代码:
```
#include <stdio.h>
#include <string.h>
typedef struct {
int id; // 商品编号
char name[50]; // 商品名
float price; // 单价
int quantity; // 数量
} Product;
int main() {
Product products[100]; // 定义包含 100 个商品的结构体数组
// 循环读入每个商品的数据
for (int i = 0; i < 100; i++) {
printf("请输入第 %d 个商品的信息:\n", i + 1);
printf("商品编号:");
scanf("%d", &products[i].id);
printf("商品名:");
scanf("%s", products[i].name);
printf("单价:");
scanf("%f", &products[i].price);
printf("数量:");
scanf("%d", &products[i].quantity);
}
// 循环遍历每个商品,计算商品总价并输出
for (int i = 0; i < 100; i++) {
float total_price = products[i].price * products[i].quantity;
printf("商品编号:%d,商品名:%s,单价:%.2f,数量:%d,总价:%.2f\n",
products[i].id, products[i].name, products[i].price,
products[i].quantity, total_price);
}
return 0;
}
```
在上面的代码中,我们首先定义了包含 "商品编号"、"商品名"、"单价" 和 "数量" 的结构体,并使用 typedef 定义了一个别名 Product。然后,我们在 main 函数中定义了一个包含 100 个商品的结构体数组 products。
接下来,我们使用一个 for 循环读入每个商品的数据。在循环中,我们使用 scanf 函数分别读入每个商品的 "商品编号"、"商品名"、"单价" 和 "数量"。
最后,我们再使用一个 for 循环遍历每个商品,计算商品总价并输出到控制台上。
希望这个程序能够帮到你!
相关推荐
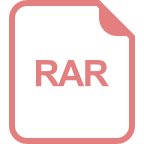
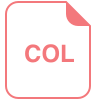
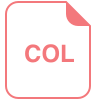
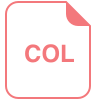
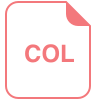
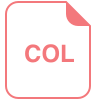









