c语言实现线程池
时间: 2023-06-29 09:17:10 浏览: 118
好的,下面是一个简单的C语言实现线程池的例子:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define THREAD_NUM 5
#define QUEUE_SIZE 10
typedef struct {
void (*function)(void *);
void *argument;
} task_t;
typedef struct {
task_t *queue;
int front, rear;
int size;
int count;
pthread_mutex_t lock;
pthread_cond_t not_empty;
pthread_cond_t not_full;
} thread_pool_t;
void *worker_thread(void *arg) {
thread_pool_t *pool = (thread_pool_t *)arg;
task_t task;
while (1) {
pthread_mutex_lock(&(pool->lock));
while (pool->count == 0) {
pthread_cond_wait(&(pool->not_empty), &(pool->lock));
}
task = pool->queue[pool->front];
pool->front = (pool->front + 1) % pool->size;
pool->count--;
pthread_cond_signal(&(pool->not_full));
pthread_mutex_unlock(&(pool->lock));
(*(task.function))(task.argument);
}
pthread_exit(NULL);
}
void initialize_thread_pool(thread_pool_t *pool) {
pthread_mutex_init(&(pool->lock), NULL);
pthread_cond_init(&(pool->not_empty), NULL);
pthread_cond_init(&(pool->not_full), NULL);
pool->queue = (task_t *)malloc(sizeof(task_t) * QUEUE_SIZE);
pool->front = 0;
pool->rear = -1;
pool->size = QUEUE_SIZE;
pool->count = 0;
int i;
for (i = 0; i < THREAD_NUM; i++) {
pthread_t tid;
pthread_create(&tid, NULL, worker_thread, (void *)pool);
}
}
void add_task(thread_pool_t *pool, void (*function)(void *), void *argument) {
pthread_mutex_lock(&(pool->lock));
while (pool->count == pool->size) {
pthread_cond_wait(&(pool->not_full), &(pool->lock));
}
pool->rear = (pool->rear + 1) % pool->size;
pool->queue[pool->rear].function = function;
pool->queue[pool->rear].argument = argument;
pool->count++;
pthread_cond_signal(&(pool->not_empty));
pthread_mutex_unlock(&(pool->lock));
}
int main() {
thread_pool_t pool;
initialize_thread_pool(&pool);
// add tasks to the thread pool
// ...
return 0;
}
```
这个例子中,我们实现了一个线程池,其中包含了一个任务队列和若干个工作线程。当有新的任务加入到队列中时,会有一个工作线程从队列中取出任务并执行。如果队列已满或者队列中没有任务,则等待条件变量的信号。在这个例子中,我们使用了pthread库来创建线程和互斥锁,以及条件变量来控制线程的同步。
阅读全文
相关推荐
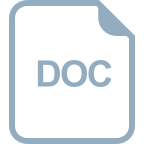
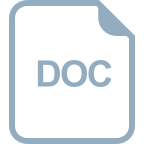
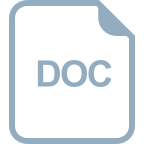
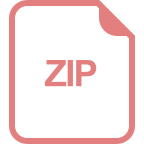
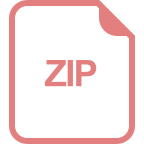
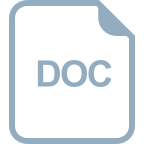
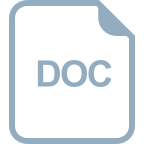
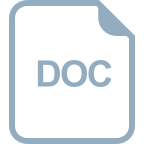
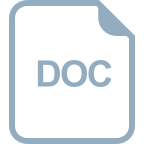




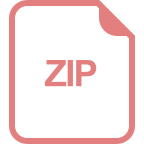
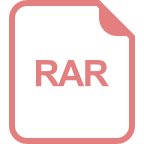
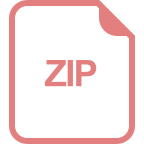
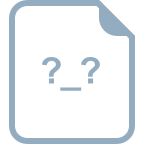