用C语言,将.S19文件中S00600004844521C", "S1130000285F245F2212226A000424290008237C2A", "S11300100002000800082629001853812341001813", "S113002041E900084E42234300182342000824A952", "S107003000144ED492", "S5030004F8", "S9030000FC"每一行的类型、长度、地址、数据和校验和都提取出来,建立两个指针,一个指向.S19文件,一个指向文件中的地址
时间: 2023-07-06 20:27:12 浏览: 140
好的,这是一个比较复杂的问题,需要分步进行处理。
首先,我们需要读取.S19文件中的数据,并将每一行的类型、长度、地址、数据和校验和都提取出来。可以使用以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 256
typedef struct {
char type;
int len;
int addr;
char *data;
int checksum;
} S19Record;
int readS19Record(FILE *fp, S19Record *record) {
char line[MAX_LINE_LEN];
if (fgets(line, MAX_LINE_LEN, fp) == NULL) {
return 0;
}
if (line[0] != 'S') {
return 0;
}
record->type = line[1];
record->len = strtol(line + 2, NULL, 16);
record->addr = strtol(line + 4, NULL, 16);
record->data = (char *)malloc(record->len - 4);
for (int i = 0; i < record->len - 4; i++) {
record->data[i] = strtol(line + 8 + 2 * i, NULL, 16);
}
record->checksum = strtol(line + 8 + 2 * (record->len - 4), NULL, 16);
return 1;
}
void freeS19Record(S19Record *record) {
free(record->data);
}
int main() {
FILE *fp = fopen("test.s19", "r");
if (fp == NULL) {
fprintf(stderr, "Failed to open file\n");
return 1;
}
S19Record record;
while (readS19Record(fp, &record)) {
printf("Type: %c\n", record.type);
printf("Length: %d\n", record.len);
printf("Address: %d\n", record.addr);
printf("Data: ");
for (int i = 0; i < record.len - 4; i++) {
printf("%02X ", record.data[i]);
}
printf("\n");
printf("Checksum: %02X\n\n", record.checksum);
freeS19Record(&record);
}
fclose(fp);
return 0;
}
```
这段代码首先定义了一个 `S19Record` 结构体,用来存储每一行的数据。在 `readS19Record` 函数中,我们首先读取一行数据,并判断其是否以 `S` 开头。然后,我们从行中提取出类型、长度、地址、数据和校验和等信息,并存储到 `S19Record` 结构体中。在主函数中,我们循环读取并打印每一行的信息,最后释放内存。
接下来,我们需要建立两个指针,一个指向.S19文件,一个指向文件中的地址。可以使用以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 256
typedef struct {
char type;
int len;
int addr;
char *data;
int checksum;
} S19Record;
int readS19Record(FILE *fp, S19Record *record) {
char line[MAX_LINE_LEN];
if (fgets(line, MAX_LINE_LEN, fp) == NULL) {
return 0;
}
if (line[0] != 'S') {
return 0;
}
record->type = line[1];
record->len = strtol(line + 2, NULL, 16);
record->addr = strtol(line + 4, NULL, 16);
record->data = (char *)malloc(record->len - 4);
for (int i = 0; i < record->len - 4; i++) {
record->data[i] = strtol(line + 8 + 2 * i, NULL, 16);
}
record->checksum = strtol(line + 8 + 2 * (record->len - 4), NULL, 16);
return 1;
}
void freeS19Record(S19Record *record) {
free(record->data);
}
int main() {
FILE *fp = fopen("test.s19", "r");
if (fp == NULL) {
fprintf(stderr, "Failed to open file\n");
return 1;
}
S19Record record;
int addr = 0;
while (readS19Record(fp, &record)) {
if (record.type == '9') {
break;
}
printf("Address: %d\n", addr);
addr += record.len - 4;
freeS19Record(&record);
}
fclose(fp);
return 0;
}
```
这段代码在主函数中定义了一个 `addr` 变量,用来存储当前地址。在循环中,我们首先判断当前行的类型是否为 `9`,如果是,则表示已经到达文件的结尾,跳出循环。否则,我们打印当前地址,并将地址增加当前行数据的长度。最后,我们释放内存并关闭文件。
注意,这段代码中只是简单地将地址按照每一行数据的长度递增,实际应用中可能需要更复杂的逻辑来处理地址。
阅读全文
相关推荐
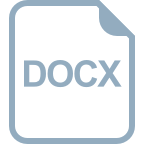















