WPF怎么讲将image转化为 流
时间: 2024-09-19 17:10:24 浏览: 40
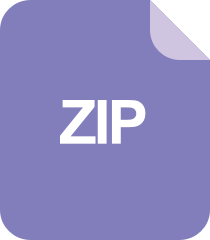
WPF播放实时视频流
在WPF (Windows Presentation Foundation) 中,如果你想把一个 `Image` 控件的内容转换成流(Stream),可以按照以下步骤操作:
1. 首先,获取 `Image` 的 `Source` 属性,这通常是一个 `ImageSource` 对象,如 `BitmapImage` 或者从文件、网络加载的资源。
```csharp
var imageSource = myImage.Source as BitmapImage;
```
2. 如果 `imageSource` 是 `BitmapImage` 类型,你可以直接调用其 `Save` 方法,传入一个 `Stream` 参数来保存图片内容。例如,保存到内存流:
```csharp
using (MemoryStream ms = new MemoryStream())
{
imageSource.Save(ms, System.Windows.Media.Imaging.ImageFormat.Png); // 可选择不同的图像格式
byte[] imageData = ms.ToArray();
}
```
3. 现在 `imageData` 就包含了图片的数据,你可以将其作为字节数组使用,或者进一步处理、传输或存储。
注意:如果 `ImageSource` 是其他类型的源,比如 `UriSource`,那么需要先加载到 `BitmapImage` 才能转换。
阅读全文
相关推荐
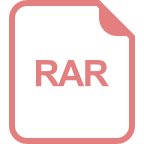
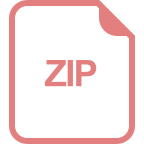


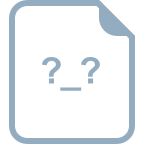
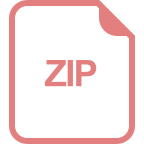
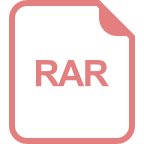
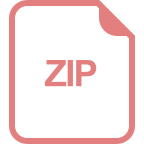
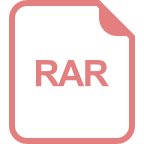
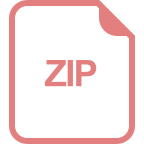
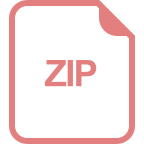
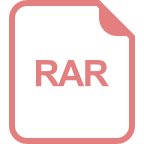
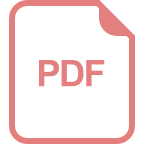
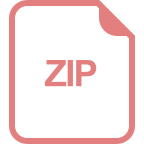
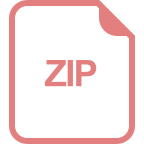
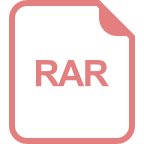

