spring boot整合redis
时间: 2023-08-31 11:35:17 浏览: 188
### 回答1:
好的,关于springboot整合redis的问题,我可以回答。SpringBoot是一种基于Spring框架的快速开发框架,可以快速集成各种组件,其中包括Redis。要整合Redis,需要在SpringBoot项目的pom.xml文件中添加Redis的依赖,同时在配置文件中添加Redis的配置信息。具体的步骤可以参考SpringBoot官方文档或者一些在线教程。
### 回答2:
Spring Boot整合Redis可以通过以下步骤实现:
1. 首先,在pom.xml文件中添加Redis相关的依赖项。可以使用Spring Data Redis依赖(spring-boot-starter-data-redis)和Jedis依赖(jedis)。
2. 创建Redis的配置文件,可以在application.properties或application.yml中配置Redis的主机名、端口号、密码等信息。
3. 创建一个配置类,用于配置Redis连接工厂和Redis模板。可以通过使用`@Configuration`注解和`redisConnectionFactory()`方法来配置Redis连接工厂,通过使用`@Bean`注解和`redisTemplate()`方法来配置Redis模板。
4. 创建一个服务类,用于实现对Redis的操作。可以使用Spring Data Redis提供的RedisTemplate类来实现常见的操作,如存储数据、获取数据、删除数据等。
5. 在需要使用Redis的地方,可以通过依赖注入的方式来使用Redis服务。
以下是一个简单的示例代码:
1. pom.xml文件中添加依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
</dependency>
```
2. application.properties中配置Redis信息:
```
spring.redis.host=127.0.0.1
spring.redis.port=6379
spring.redis.password=
```
3. 创建Redis的配置类:
```java
@Configuration
public class RedisConfig {
@Bean
JedisConnectionFactory redisConnectionFactory() {
RedisStandaloneConfiguration redisStandaloneConfiguration = new RedisStandaloneConfiguration();
redisStandaloneConfiguration.setHostName("127.0.0.1");
redisStandaloneConfiguration.setPort(6379);
return new JedisConnectionFactory(redisStandaloneConfiguration);
}
@Bean
public RedisTemplate<String, Object> redisTemplate() {
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory());
return template;
}
}
```
4. 创建Redis的服务类:
```java
@Service
public class RedisService {
@Autowired
private RedisTemplate<String, Object> redisTemplate;
public void setValue(String key, Object value) {
redisTemplate.opsForValue().set(key, value);
}
public Object getValue(String key) {
return redisTemplate.opsForValue().get(key);
}
public void deleteValue(String key) {
redisTemplate.delete(key);
}
}
```
5. 在需要使用Redis的地方,可以通过依赖注入RedisService来使用Redis服务:
```java
@RestController
public class ExampleController {
@Autowired
private RedisService redisService;
@GetMapping("/example")
public String example() {
redisService.setValue("name", "John");
String name = (String) redisService.getValue("name");
redisService.deleteValue("name");
return name;
}
}
```
通过以上步骤,就可以在Spring Boot应用中使用Redis进行数据存储、获取和删除等操作了。
### 回答3:
Spring Boot是一个用于创建和构建Spring应用程序的开源框架。它简化了Spring应用程序的开发过程,并提供了各种开箱即用的功能和集成。Redis是一个流行的用于缓存和存储数据的内存数据库。下面是如何在Spring Boot应用程序中整合Redis的步骤:
1. 添加依赖:在项目的pom.xml文件中添加Redis的依赖项。可以使用Spring Boot提供的spring-boot-starter-data-redis依赖项。在构建工具Maven中,可以通过以下方式添加依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
2. 配置Redis连接:在Spring Boot的配置文件中,配置Redis的连接信息,包括主机名、端口号、密码等。可以使用如下配置示例:
```properties
spring.redis.host=localhost
spring.redis.port=6379
spring.redis.password=
```
3. 创建RedisTemplate bean:在Spring Boot的配置类中,创建RedisTemplate的bean。RedisTemplate是Spring提供的用于与Redis进行交互的工具类。可以使用如下方式创建bean:
```java
@Configuration
public class RedisConfig {
@Value("${spring.redis.host}")
private String host;
@Value("${spring.redis.port}")
private int port;
@Value("${spring.redis.password}")
private String password;
@Bean
public RedisConnectionFactory redisConnectionFactory() {
RedisStandaloneConfiguration config = new RedisStandaloneConfiguration(host, port);
config.setPassword(RedisPassword.of(password));
return new LettuceConnectionFactory(config);
}
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
RedisTemplate<String, Object> redisTemplate = new RedisTemplate<>();
redisTemplate.setConnectionFactory(redisConnectionFactory);
return redisTemplate;
}
}
```
4. 使用RedisTemplate:在需要使用Redis的地方,注入RedisTemplate并使用其提供的方法来操作Redis。例如,可以在服务类中使用RedisTemplate进行数据的读取和写入操作:
```java
@Service
public class UserService {
@Autowired
private RedisTemplate<String, Object> redisTemplate;
public User getUserById(String userId) {
String key = "user:" + userId;
return (User) redisTemplate.opsForValue().get(key);
}
public void saveUser(User user) {
String key = "user:" + user.getId();
redisTemplate.opsForValue().set(key, user);
}
}
```
通过以上步骤,就可以在Spring Boot应用程序中成功地整合Redis,并通过RedisTemplate进行数据的读写操作。这样可以更加方便地利用Redis的优势来提升应用程序的性能和可扩展性。
阅读全文
相关推荐
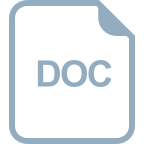
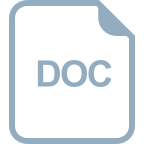
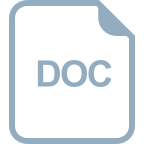
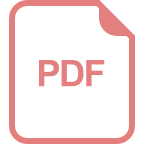
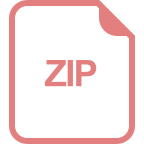


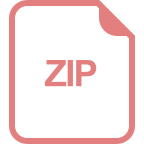
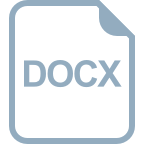
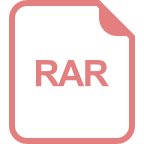
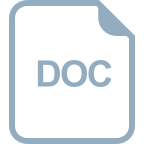





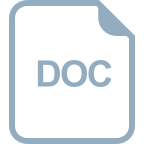
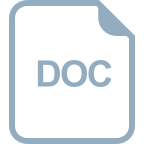
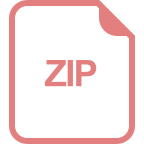