解释下面这段代码public class Maze { //初始化一个地图 默认所有路不通 //最终产生的二维数组大小实际为(2width+1) * (2height+1) private static int width;//宽度 private static int height;//高度 public static int[][] map;// 存放迷宫的数组 private static int rows;//行数 private stati
时间: 2024-01-05 10:03:27 浏览: 90
这段代码定义了一个Maze类,用于生成一个迷宫地图。其中:
- `private static int width;` 和 `private static int height;` 定义了地图的宽度和高度。
- `public static int[][] map;` 定义了一个二维数组用于存放迷宫地图。
- `private static int rows;` 和 `private static int cols;` 定义了地图数组的行数和列数,实际上是 `(2width+1)` 和 `(2height+1)`。
- `public Maze(int w, int h)` 是类的构造函数,用于初始化地图大小和数组。
在构造函数中,首先根据传入的宽度和高度计算出地图数组的行数和列数,然后初始化地图数组 `map`,并将所有元素初始化为0,表示所有的路都不通。最后,使用 `generateMaze()` 方法生成迷宫地图。
相关问题
Java假定用二维数组表示迷宫,墙用1表示,通路用0表示。迷宫的左上角为起点,假设坐标(1,1),迷宫的终点右下角,坐标为(8,8)。请使用栈,找出一条从起点到终点的走迷宫路线并输出此路线上每个点的坐标。
在Java中,可以使用深度优先搜索(DFS)算法结合栈来解决这个问题。以下是使用二维数组和栈实现的一种解决方案:
```java
import java.util.ArrayList;
import java.util.List;
class Point {
int x;
int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
@Override
public String toString() {
return "(" + x + ", " + y + ")";
}
}
public class MazeSolver {
private static final int[][] MAZE = {{0, 0, 1, 1, 0, 0},
{0, 0, 0, 0, 1, 0},
{1, 1, 0, 0, 1, 1},
{1, 1, 0, 0, 0, 0},
{1, 1, 1, 0, 0, 0},
{0, 0, 0, 0, 0, 0}};
public static List<Point> findPath(int startX, int startY, int endX, int endY) {
List<Point> path = new ArrayList<>();
Stack<Point> stack = new Stack<>();
stack.push(new Point(startX, startY));
path.add(new Point(startX, startY));
while (!stack.isEmpty()) {
Point current = stack.pop();
// 检查当前位置是否是终点
if (current.x == endX && current.y == endY) {
break;
}
// 邻居遍历,将可达的非墙位置压入栈
for (int dx = -1; dx <= 1; dx++) {
for (int dy = -1; dy <= 1; dy++) {
int nx = current.x + dx;
int ny = current.y + dy;
// 只有当移动到的坐标在合法范围内,并且不是墙的位置才继续
if (nx >= 0 && nx < MAZE.length && ny >= 0 && ny < MAZE[0].length && MAZE[nx][ny] == 0) {
stack.push(new Point(nx, ny));
path.add(new Point(nx, ny));
}
}
}
}
return path;
}
public static void main(String[] args) {
List<Point> path = findPath(1, 1, 8, 8);
if (path.isEmpty()) {
System.out.println("无法从起点到达终点");
} else {
for (Point point : path) {
System.out.println(point);
}
}
}
}
```
这个程序首先初始化一个起点(1,1),并将它放入栈中。然后不断弹出栈顶元素,检查其是否为目标点,如果不是,则遍历所有相邻的点(上下左右)。如果是非墙位置并且未访问过,将其加入路径列表并压入栈。最后,返回找到的路径。
注意,如果没有找到路径,`findPath`方法会返回一个空列表。在`main`函数中,我们打印路径中的每个点。
写一段VC++迷宫MFC的代码
在VC++中创建一个基本的MFC(Microsoft Foundation Classes)迷宫游戏,我们通常会使用CFrameWindow作为基础窗口,并结合CView和CMFCGridCtrl来显示和交互迷宫。这里是一个简单的示例,展示如何在一个窗口上绘制一个二维迷宫:
```cpp
// MFC迷宫窗口头文件(CMazeGame.h)
#pragma once
#include "resource.h"
#include <vector>
#include <atlheaders.h>
class CMazeGame : public CFrameWnd
{
public:
enum { IDD = IDD_MAIZEGAME };
CMazeGame();
protected:
virtual void OnInitialUpdate(); // Override to perform any initial creation of controls
private:
CButton* m_pButtonStart;
CButton* m_pButtonEnd;
std::vector<std::vector<bool>> m_Maze; // 真值数组表示墙(0)和通道(1)
DECLARE_MESSAGE_MAP()
};
// MFC迷宫窗口实现文件(CMazeGame.cpp)
#include "CMazeGame.h"
CMazeGame::CMazeGame()
{
// 初始化迷宫矩阵...
Create(NULL, L"Maze Game", NULL, WS_OVERLAPPEDWINDOW | FWS_ADDTOTITLE,
CRect(0, 0, 400, 300), nullptr, nullptr);
}
void CMazeGame::OnInitialUpdate()
{
CDialogBar* pDlgBar = GetDlgItemBar(this, AFX_IDW_DIALOGBAR);
if (pDlgBar)
{
m_pButtonStart = reinterpret_cast<CButton*>(pDlgBar->FindChild(IDC_START));
m_pButtonEnd = reinterpret_cast<CButton*>(pDlgBar->FindChild(IDC_END));
// 添加绘图处理...
CMFCGridCtrl& gridCtrl = GetDlgItem(IDC_GRID);
for (int i = 0; i < m_Maze.size(); i++)
for (int j = 0; j < m_Maze[i].size(); j++) {
if (m_Maze[i][j]) {
// 绘制墙壁
CPen pen(HOLLOW_BLACK_PEN);
gridCtrl.SetPen(&pen);
gridCtrl.DrawRect(CRect(j * 50, i * 50, (j + 1) * 50 - 1, (i + 1) * 50 - 1));
}
}
}
// 调用基类方法完成初始更新
CFrameWnd::OnInitialUpdate();
}
BEGIN_MESSAGE_MAP(CMazeGame, CFrameWnd)
ON_WM_PAINT() // 画布事件处理
ON_BN_CLICKED(IDC_START, &CMazeGame::OnBnClickedStart) // 开始按钮点击事件
ON_BN_CLICKED(IDC_END, &CMazeGame::OnBnClickedEnd) // 结束按钮点击事件
END_MESSAGE_MAP()
// ...其他事件处理函数...
```
在这个例子中,你需要定义`m_Maze`矩阵来设置迷宫布局,并在OnPaint方法中添加绘画逻辑。当用户点击开始或结束按钮时,可以触发相应的游戏逻辑。
阅读全文
相关推荐
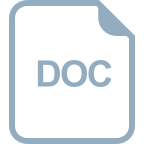
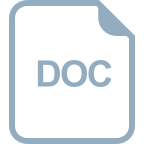
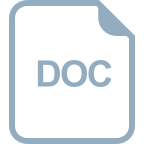
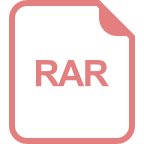
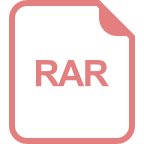
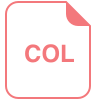
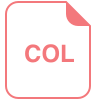
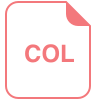
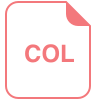
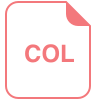






