用python做个浏览器
时间: 2023-09-02 08:14:21 浏览: 151
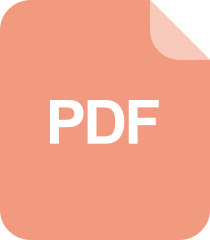
使用python调用浏览器并打开一个网址的例子
要用 Python 实现一个浏览器,需要用到以下几个模块:
1. `urllib` 模块:用于对 URL 进行解析和处理。
2. `socket` 模块:用于创建 TCP 连接和发送 HTTP 请求。
3. `re` 模块:用于正则表达式匹配。
4. `tkinter` 模块:用于 GUI 界面的开发。
下面是一个简单的 Python 浏览器的代码示例:
```python
import urllib.request
import socket
import re
import tkinter as tk
class Browser:
def __init__(self, root):
self.root = root
self.root.title("Python Browser")
self.url_entry = tk.Entry(self.root)
self.url_entry.pack(fill=tk.X)
self.go_button = tk.Button(self.root, text="Go", command=self.fetch_url)
self.go_button.pack()
self.html_text = tk.Text(self.root)
self.html_text.pack(fill=tk.BOTH, expand=True)
def fetch_url(self):
url = self.url_entry.get()
if not url.startswith("http://") and not url.startswith("https://"):
url = "http://" + url
try:
response = urllib.request.urlopen(url)
html = response.read().decode()
self.html_text.delete(1.0, tk.END)
self.html_text.insert(tk.END, html)
except (urllib.error.URLError, socket.timeout):
self.html_text.delete(1.0, tk.END)
self.html_text.insert(tk.END, "Failed to fetch URL.")
root = tk.Tk()
browser = Browser(root)
root.mainloop()
```
这个浏览器使用 tkinter 模块创建了一个简单的界面,包括一个输入框、一个按钮和一个文本框。当用户点击按钮后,浏览器将使用 urllib 和 socket 模块发送 HTTP 请求并获取响应,然后将响应的 HTML 内容显示在文本框中。
阅读全文
相关推荐
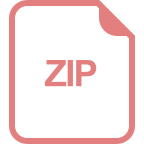
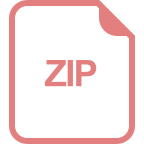

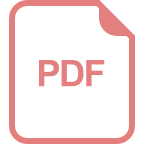
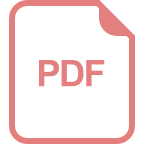
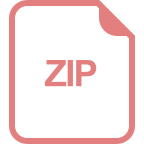
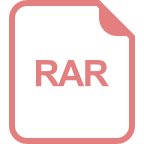
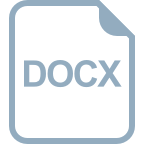
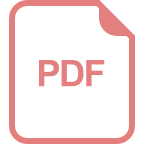
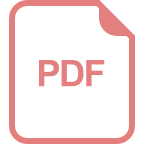
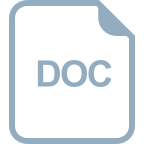
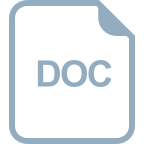


