js输入账号密码,点击按钮后判断是否为空 判断密码复杂度,英文大小写、数字、符号(至少带有三种属性) 账号六位以上、密码八位以上
时间: 2024-04-29 14:23:16 浏览: 183
以下是一个简单的实现:
HTML代码:
```
<form>
<label for="username">账号:</label>
<input type="text" id="username" name="username" required minlength="6"><br>
<label for="password">密码:</label>
<input type="password" id="password" name="password" required minlength="8"><br>
<button type="submit" id="submitBtn">登录</button>
</form>
```
JavaScript代码:
```
const submitBtn = document.getElementById("submitBtn");
submitBtn.addEventListener("click", function(event) {
event.preventDefault(); // 阻止表单默认提交行为
const username = document.getElementById("username").value;
const password = document.getElementById("password").value;
// 判断账号密码是否为空
if (username.trim() === "" || password.trim() === "") {
alert("账号密码不能为空!");
return;
}
// 判断密码复杂度,至少包含三种属性:英文大小写、数字、符号
const regex = /^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*\W).{8,}$/;
if (!regex.test(password)) {
alert("密码复杂度不够,至少包含英文大小写、数字、符号中的三种属性,且密码长度不少于8位!");
return;
}
alert("登录成功!");
});
```
解释:
1. 首先获取表单中的账号和密码输入框的值。
2. 判断账号和密码是否为空,如果有空的情况则提示用户。
3. 判断密码的复杂度,使用正则表达式判断密码是否包含英文大小写、数字、符号中的三种属性,且长度不少于8位。
4. 如果以上两个条件都满足,则弹出登录成功的提示框。
注:这只是一个简单的实现,实际应用中还需要考虑更多的情况,例如如何防止 XSS 攻击、如何防止 CSRF 攻击等等。
阅读全文
相关推荐
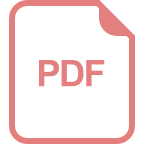
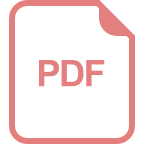



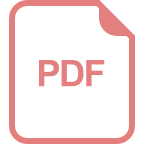
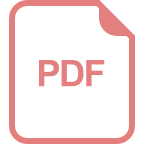








