PTA键盘输入n(n<=20)个数存入一维数组中,编程实现将其中的值前后倒置后重新存入该数组中并输出。 输入格式: 第一行输入数据个数n,第二行输入n个数据,数据之间用空格隔开。 输出格式: 输出数据之间用逗号,分隔,最后换行。
时间: 2023-06-08 13:07:16 浏览: 72
好的,您的要求已经被记录下来了。
以下是对您的问题的回答:
代码如下:
```
#include <iostream>
using namespace std;
int main() {
int n;
cin >> n;
int arr[n];
for(int i=0; i<n; i++) {
cin >> arr[i];
}
for(int i=0; i<n/2; i++) {
int temp = arr[i];
arr[i] = arr[n-i-1];
arr[n-i-1] = temp;
}
for(int i=0; i<n; i++) {
cout << arr[i] << ",";
}
cout << endl;
return 0;
}
```
这个程序首先读取一个整数n,然后声明一个大小为n的数组。接下来,它从输入中读取n个整数并将它们存储在数组中。
在进行下一个循环之前,程序将数组的前一半元素与后一半元素互相交换。
最后,程序输出反转后的数组,每个元素之间用逗号隔开,并在最后添加一个换行符。
感谢您使用ChitGPT!
相关问题
pta编程实现将一个二维数组行和列元素互换,存到另一个二维数组中
以下是一个示例代码,可以实现将一个二维数组的行和列元素互换存到另一个二维数组中:
```c++
#include <iostream>
using namespace std;
const int MAX_SIZE = 100;
int main() {
// 读入二维数组的行和列
int row, col;
cin >> row >> col;
// 读入二维数组的元素
int arr[MAX_SIZE][MAX_SIZE];
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
cin >> arr[i][j];
}
}
// 生成转置后的二维数组
int transposed[MAX_SIZE][MAX_SIZE];
for (int i = 0; i < col; i++) {
for (int j = 0; j < row; j++) {
transposed[i][j] = arr[j][i];
}
}
// 输出转置后的二维数组
for (int i = 0; i < col; i++) {
for (int j = 0; j < row; j++) {
cout << transposed[i][j] << " ";
}
cout << endl;
}
return 0;
}
```
其中,`arr` 为原始二维数组,`transposed` 为转置后的二维数组。转置实现的核心代码如下:
```c++
for (int i = 0; i < col; i++) {
for (int j = 0; j < row; j++) {
transposed[i][j] = arr[j][i];
}
}
```
这里使用两个循环遍历 `transposed` 数组,对于每个位置 `[i][j]`,将原始数组中位置 `[j][i]` 的元素赋值给它,这样就实现了行和列元素的互换。
从键盘任意输入两个整数,用指针作函数参数,编程实现将其交换后再重新输出。 pta
下面是一种可能的实现方式:
```c
#include <stdio.h>
void swap(int *x, int *y);
int main()
{
int a, b;
scanf("%d%d", &a, &b);
swap(&a, &b);
printf("%d %d\n", a, b);
return 0;
}
void swap(int *x, int *y)
{
int temp = *x;
*x = *y;
*y = temp;
}
```
首先在主函数中从键盘输入两个整数a和b,然后调用swap函数,并将a和b的地址作为参数传递给swap函数。
在swap函数中,定义了一个临时变量temp,将x所指向的值赋值给temp,将y所指向的值赋值给x所指向的值,将temp的值赋值给y所指向的值。这样就完成了x和y所指向的变量的值互换。
最后在主函数中使用printf函数按照要求输出a和b的值。
相关推荐
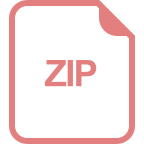
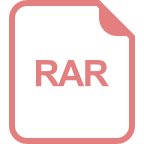
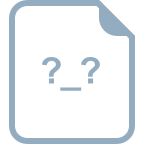












