python def func ->
时间: 2023-07-21 07:11:44 浏览: 47
In Python, `def` is a keyword used to define a function. The basic syntax of defining a function is:
```
def function_name(parameters):
"""docstring"""
# code block
return [expression]
```
Here, `function_name` is the name of the function, and `parameters` are the inputs that the function takes. The code block under the function definition is executed when the function is called, and the `return` statement specifies the value that the function returns (if any).
The `docstring` is a string literal that describes what the function does. It is optional, but it is good practice to include informative docstrings in your code to help others understand how to use your function.
相关问题
Python---闭包
闭包是指在函数内部定义的函数,该函数可以访问外部函数的变量,并且可以在外部函数调用结束后继续存在。闭包常用于实现装饰器、工厂函数等。
下面是一个简单的闭包示例:
```python
def outer_func(x):
def inner_func(y):
return x + y
return inner_func
add_five = outer_func(5)
print(add_five(3)) # 输出 8
```
在上面的示例中,`outer_func` 是一个外部函数,它接受一个参数 `x`,并返回一个内部函数 `inner_func`。`inner_func` 接受一个参数 `y`,并返回 `x + y` 的结果。在调用 `outer_func(5)` 后,我们得到了一个新的函数 `add_five`,它是 `inner_func` 的一个实例,并且 `x` 被设置为了 5。因此,当我们调用 `add_five(3)` 时,它返回了 8。
Python中func用法
在Python中,func通常是函数的缩写,用于表示一个可调用的对象。函数是一种在Python中定义可重复使用代码的方式。
以下是Python中func的用法示例:
1. 定义一个函数:
```python
def my_func(x, y):
return x + y
```
2. 将函数作为参数传递给另一个函数:
```python
def apply(func, x, y):
return func(x, y)
result = apply(my_func, 2, 3) # result = 5
```
3. 使用lambda表达式创建一个函数:
```python
lambda_func = lambda x: x * 2
result = lambda_func(2) # result = 4
```
4. 将函数作为返回值:
```python
def create_adder(x):
def adder(y):
return x + y
return adder
add_5 = create_adder(5)
result = add_5(3) # result = 8
```
这些是Python中func的常见用法示例。
相关推荐
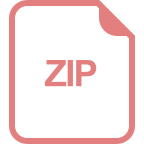
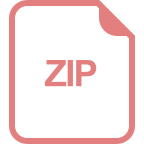
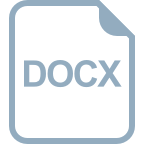












