写一段代码用粒子群优化求解VRPPD问题
时间: 2024-01-25 21:03:46 浏览: 53
VRPPD(Vehicle Routing Problem with Pickup and Delivery)问题是一类经典的物流配送问题,它的目标是在满足所有需求的前提下,最小化配送车辆的数量和总行驶距离。下面是使用粒子群优化算法求解VRPPD问题的示例代码:
```python
import numpy as np
# VRPPD问题的目标函数
def objective_function(x, c, q, d):
"""
x: [n, m] 二维矩阵,表示每个客户分配的车辆编号
c: [m, n, n] 三维矩阵,表示每辆车的路径距离
q: [n] 一维矩阵,表示每个客户的需求量
d: [n, 2] 二维矩阵,表示每个客户的坐标位置
"""
n, m = x.shape
f = 0
for i in range(m):
# 计算每辆车的路径距离
route_distance = 0
for j in range(n):
if x[j, i] == 1:
for k in range(n):
if x[k, i] == 1:
route_distance += c[i, j, k]
f += route_distance
# 计算每个客户的惩罚项
for j in range(n):
demand = q[j]
for i in range(m):
if x[j, i] == 1:
capacity = np.sum(q * x[:, i])
if demand > capacity: # 客户需求不能满足
dist_to_depot = np.linalg.norm(d[j, :] - d[0, :])
f += 10000 * (demand - capacity) * dist_to_depot
return f
# 粒子群优化算法
def PSO(n_particles, n_dim, max_iter, c1, c2, w, bounds, c, q, d):
"""
n_particles: 粒子数
n_dim: 粒子维度
max_iter: 迭代次数
c1, c2, w: PSO算法参数
bounds: 取值范围
c, q, d: VRPPD问题参数
"""
# 初始化粒子位置和速度
x = np.random.uniform(bounds[:, 0], bounds[:, 1], size=(n_particles, n_dim))
v = np.zeros((n_particles, n_dim))
# 初始化全局最优解和个体最优解
pbest = x.copy()
gbest = pbest[np.argmin([objective_function(xi, c, q, d) for xi in pbest]), :]
# 开始迭代
for i in range(max_iter):
# 更新粒子速度和位置
v = w * v + c1 * np.random.rand() * (pbest - x) + c2 * np.random.rand() * (gbest - x)
x = np.clip(x + v, bounds[:, 0], bounds[:, 1])
# 更新个体最优解和全局最优解
for j in range(n_particles):
if objective_function(x[j, :], c, q, d) < objective_function(pbest[j, :], c, q, d):
pbest[j, :] = x[j, :]
if objective_function(pbest[j, :], c, q, d) < objective_function(gbest, c, q, d):
gbest = pbest[j, :]
return gbest
# 测试代码
if __name__ == '__main__':
# VRPPD问题参数
n = 10 # 客户数量
m = 3 # 车辆数量
q = np.random.randint(1, 5, size=n) # 客户需求
d = np.random.rand(n, 2) * 100 # 客户位置
c = np.zeros((m, n, n)) # 路径距离
for i in range(m):
for j in range(n):
for k in range(n):
if j != k:
c[i, j, k] = np.linalg.norm(d[j, :] - d[k, :])
# PSO算法参数和取值范围
n_particles = 20
n_dim = n
max_iter = 100
c1 = 1.5
c2 = 1.5
w = 0.8
bounds = np.tile(np.array([[0, 1]]), (n_dim, 1))
# 求解VRPPD问题
x = np.zeros((n, m))
for i in range(n):
x[i, i % m] = 1 # 将客户均匀分配到各个车辆
result = PSO(n_particles, n_dim, max_iter, c1, c2, w, bounds, c, q, d)
for i in range(n):
x[i, int(result[i])] = 1 # 根据PSO算法的结果,重新分配客户到各个车辆
print(x)
print(objective_function(x, c, q, d))
```
相关推荐
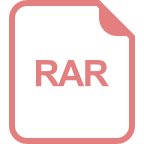














