java创建一个可以放置九张图像的窗口,用按钮切换图像
时间: 2023-05-24 11:03:06 浏览: 133
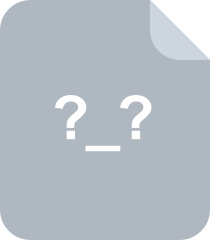
多窗口切换按钮样式设置.py
A:
可以使用Swing组件库来创建一个可以放置九张图像的窗口,并使用按钮来切换图像。下面是一个简单的Java代码示例:
```java
import java.awt.*;
import javax.swing.*;
public class ImageSwitcher extends JFrame {
private JLabel[] imageLabels;
private ImageIcon[] imageIcons;
private JButton[] buttons;
private int currentImageIndex;
public ImageSwitcher() {
super("Image Switcher");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Create image icons
imageIcons = new ImageIcon[9];
for (int i = 0; i < 9; i++) {
imageIcons[i] = new ImageIcon("image" + (i+1) + ".jpg");
}
// Create image labels
imageLabels = new JLabel[9];
for (int i = 0; i < 9; i++) {
imageLabels[i] = new JLabel(imageIcons[i]);
}
// Create buttons
buttons = new JButton[9];
for (int i = 0; i < 9; i++) {
buttons[i] = new JButton("" + (i+1));
buttons[i].addActionListener(event -> {
currentImageIndex = Integer.parseInt(event.getActionCommand()) - 1;
updateImage();
});
}
// Create main panel and add components to it
JPanel mainPanel = new JPanel(new GridLayout(3, 3));
for (int i = 0; i < 9; i++) {
mainPanel.add(imageLabels[i]);
}
// Create control panel and add buttons to it
JPanel controlPanel = new JPanel(new GridLayout(3, 3));
for (int i = 0; i < 9; i++) {
controlPanel.add(buttons[i]);
}
// Add panels to the frame
add(mainPanel, BorderLayout.CENTER);
add(controlPanel, BorderLayout.SOUTH);
// Set current image index and update the first image
currentImageIndex = 0;
updateImage();
// Set frame size and show it
setSize(600, 600);
setVisible(true);
}
private void updateImage() {
for (int i = 0; i < 9; i++) {
imageLabels[i].setVisible(i == currentImageIndex);
}
}
public static void main(String[] args) {
new ImageSwitcher();
}
}
```
这个示例程序创建了一个名为Image Switcher的窗口,其中包含9个图像和9个按钮。当用户单击按钮时,窗口会切换到相应的图像。请注意,这个示例中的图像必须存储在与程序相同的目录中,并使用image1.jpg到image9.jpg的名称。如果需要,可以使用其他名称或从其他位置加载图像。
阅读全文
相关推荐
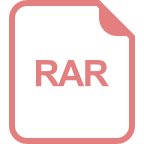
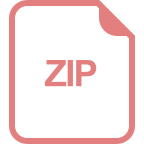
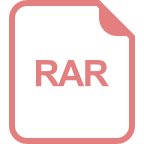
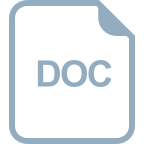
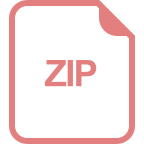
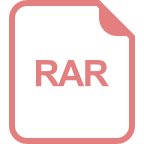
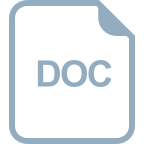
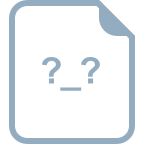
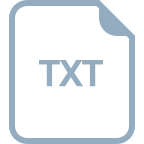
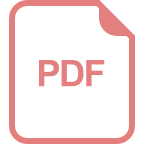
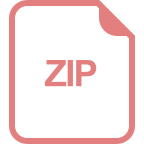
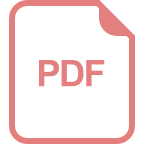
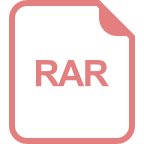
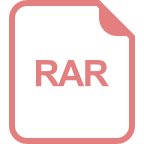
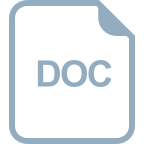
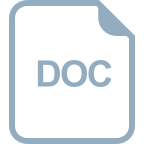