mongodb去重查询语句怎么写
时间: 2023-10-25 12:35:59 浏览: 129
使用distinct方法可以进行去重查询,语法如下:
db.collection_name.distinct("field_name")
其中,collection_name为集合名称,field_name为要进行去重的字段名称。例如,查询一个集合中所有不重复的用户性别:
db.users.distinct("gender")
相关问题
java mongodb去重查询语句
Java中使用MongoDB进行去重查询可以使用聚合管道操作来实现。以下是一个示例代码:
```java
import com.mongodb.MongoClient;
import com.mongodb.client.AggregateIterable;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
import java.util.Arrays;
public class MongoDBDistinctQuery {
public static void main(String[] args) {
// 连接到MongoDB服务器
MongoClient mongoClient = new MongoClient("localhost", 27017);
// 连接到数据库
MongoDatabase database = mongoClient.getDatabase("mydb");
// 获取集合
MongoCollection<Document> collection = database.getCollection("mycollection");
// 构建聚合操作
AggregateIterable<Document> result = collection.aggregate(Arrays.asList(
new Document("$group", new Document("_id", "$field")),
new Document("$project", new Document("_id", 0).append("distinctField", "$_id"))
));
// 遍历结果
for (Document document : result) {
System.out.println(document.get("distinctField"));
}
// 关闭连接
mongoClient.close();
}
}
```
在上述代码中,我们首先连接到MongoDB服务器,并选择要操作的数据库和集合。然后,我们使用聚合管道操作 `$group` 和 `$project` 来对指定字段进行去重操作。最后,我们遍历结果并输出去重后的字段值。
请注意,`field` 是你想要进行去重的字段名,`mydb` 是数据库名,`mycollection` 是集合名,你需要根据实际情况进行修改。
mongodb查询语句
以下是一些常用的 MongoDB 查询语句:
1. 查询所有文档:db.collection.find()
2. 根据条件查询文档:db.collection.find({条件})
3. 查询指定字段的文档:db.collection.find({},{字段1:1,字段2:1})
4. 查询指定数量的文档:db.collection.find().limit(数量)
5. 查询指定范围的文档:db.collection.find().skip(开始位置).limit(数量)
6. 查询排序的文档:db.collection.find().sort({字段:1})
7. 查询去重后的文档:db.collection.distinct({字段})
8. 查询符合条件的文档数量:db.collection.count({条件})
9. 查询并更新文档:db.collection.findAndModify({条件},{更新操作})
10. 删除符合条件的文档:db.collection.remove({条件})
阅读全文
相关推荐
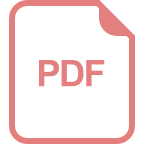
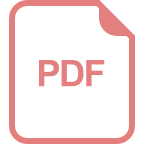
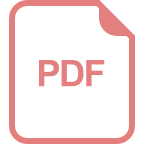
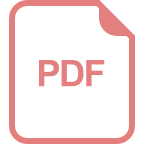
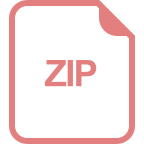
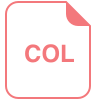
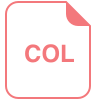
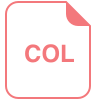
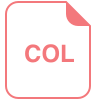



