从文件idcard.txt中读入若干个人的姓名和身份证号码,提取每个人的生日信息,将每个人的姓名和生日信息写入文件birthday.txt中。用c语言实现
时间: 2024-03-15 15:42:08 浏览: 148
好的,下面是一个使用C语言实现这个任务的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE 1000
int main()
{
FILE *fp_in, *fp_out;
char line[MAX_LINE];
char *file_in = "idcard.txt";
char *file_out = "birthday.txt";
// 打开输入文件
if ((fp_in = fopen(file_in, "r")) == NULL) {
fprintf(stderr, "Could not open input file\n");
return 1;
}
// 打开输出文件
if ((fp_out = fopen(file_out, "w")) == NULL) {
fprintf(stderr, "Could not open output file\n");
return 1;
}
// 逐行读取输入文件
while (fgets(line, MAX_LINE, fp_in) != NULL) {
// 查找生日信息
char *birthday = strstr(line, "19") // 生日信息通常以19或20开头
? strstr(line, "19")
: strstr(line, "20");
if (birthday) {
// 提取姓名和生日信息
char name[MAX_LINE];
strncpy(name, line, birthday - line);
name[birthday - line - 1] = '\0';
char birthday_str[MAX_LINE];
strncpy(birthday_str, birthday, 8);
birthday_str[8] = '\0';
// 写入输出文件
fprintf(fp_out, "%s %s\n", name, birthday_str);
}
}
// 关闭文件
fclose(fp_in);
fclose(fp_out);
return 0;
}
```
这个程序比较简单,直接查找生日信息的位置并提取即可。需要注意的是,这个程序假设生日信息在每行中只出现一次,并且格式为“年月日”,没有其他的干扰信息。如果实际情况不符合这种情况,需要根据实际情况进行修改。
阅读全文
相关推荐
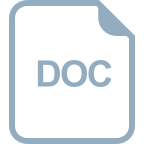
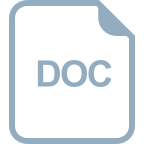
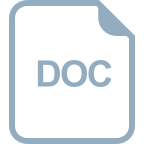





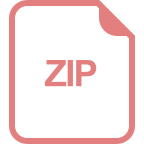
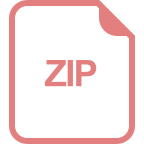
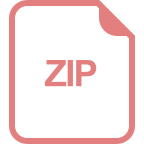
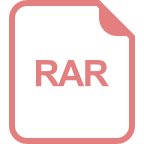



