输出月份英文名:输入月份,输出对应的英文名称。要求用指针数组表示 12 个月的英文名称。 例如,输入 5,输出 May。试编写相应程序。
时间: 2023-06-27 13:08:37 浏览: 61
下面是一个示例程序:
```c
#include <stdio.h>
int main() {
char* months[12] = {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"};
int month;
printf("Enter a month number (1-12): ");
scanf("%d", &month);
if (month >= 1 && month <= 12) {
printf("The month is %s\n", months[month-1]);
} else {
printf("Invalid month number\n");
}
return 0;
}
```
在这个程序中,我们定义了一个字符串指针数组 `months`,其中包含了 12 个月份的英文名称。然后,我们读入一个月份数字,如果数字在 1 到 12 之间,就输出对应的英文名称。注意,月份数字减 1 后对应的是 `months` 数组中的下标。
相关问题
输出月份英文名:输入月份,输出对应的英文名称。要求用指针数组表示 12 个月的英文名称。 例如,输入 5,输出 May。试用C语言编写相应程序。
好的,下面是代码实现:
```c
#include <stdio.h>
#include <string.h>
int main()
{
char *month[12] = {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"};
int input;
printf("请输入月份(1-12):");
scanf("%d", &input);
if (input < 1 || input > 12)
{
printf("无效的输入!");
}
else
{
printf("%s", *(month + input - 1));
}
return 0;
}
```
在这个程序中,我们首先定义了一个指针数组`month`,用来存储 12 个月份的英文名称。每个元素都是一个指向字符数组的指针,这个字符数组保存了对应月份的英文名称。
接着,我们通过`scanf`函数获取用户输入的月份,然后通过指针数组`month`找到对应的英文名称并输出。
需要注意的是,由于数组下标从 0 开始,而月份从 1 开始,因此需要将用户输入的月份减去 1 才能正确地访问指针数组。
输出月份英文名:输入月份,输出对应的英文名称。要求用指针数组表示 12 个月的英文名称。 例如,输入 5,输出 May。试使用c语言编写相应程序。
下面是一个简单的 C 语言程序,使用指针数组表示 12 个月份的英文名称。程序要求用户输入一个数字(1 到 12),然后输出相应的英文名称。
```c
#include <stdio.h>
int main() {
char *months[12] = {"January", "February", "March", "April",
"May", "June", "July", "August",
"September", "October", "November", "December"};
int month_number;
printf("Enter a month number (1-12): ");
scanf("%d", &month_number);
if (month_number < 1 || month_number > 12) {
printf("Invalid month number!\n");
return 1;
}
printf("The corresponding month is %s.\n", months[month_number - 1]);
return 0;
}
```
这个程序首先定义了一个指针数组 `months`,其中存储了 12 个月份的英文名称。然后程序要求用户输入一个数字,检查该数字是否在 1 到 12 之间。最后程序输出相应的英文名称。
需要注意的是,由于数组下标是从 0 开始的,因此在使用数组时需要将用户输入的数字减去 1。
相关推荐








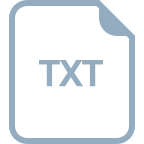
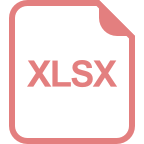
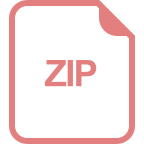