用python爬取计算机二级wps office题库代码
时间: 2023-09-09 16:05:24 浏览: 137
### 回答1:
答案:可以使用Python进行爬取计算机二级WPS Office题库的代码,主要可以使用urllib库、requests库、selenium库等抓取网页,并使用Beautifulsoup库进行网页解析,最后使用正则表达式或其他方式从网页中提取出题库信息。
### 回答2:
要使用Python爬取计算机二级WPS Office题库的代码,您需要遵循以下步骤:
第一步,导入所需的库:
```python
import requests
from bs4 import BeautifulSoup
```
第二步,发送请求并获取页面内容:
```python
url = "http://www.wps.cn/question/"
response = requests.get(url)
html_content = response.text
```
第三步,解析页面内容并提取题目信息:
```python
soup = BeautifulSoup(html_content, 'html.parser')
question_items = soup.select('.questions-item')
for item in question_items:
question = item.select_one('.subject').text.strip()
options = item.select('.options-cont .option-text')
answer = item.select_one('.answer').text.strip()
print('题目:', question)
print('选项:', [option.text.strip() for option in options])
print('答案:', answer)
print('=' * 50)
```
以上代码会将题目、选项和答案打印出来,你可以根据需要进行保存或进一步处理。
需要注意的是,这仅仅是爬取了题目的内容,并没有解决页面翻页、登录等使用问题。如果需要爬取更多题目或解决复杂的问题,可能需要进一步的代码开发。
### 回答3:
使用Python进行爬取计算机二级WPS Office题库的代码可以按照以下步骤进行:
步骤一:导入所需模块
首先,我们需要导入所需的Python模块,如requests、BeautifulSoup等。
```python
import requests
from bs4 import BeautifulSoup
```
步骤二:发送HTTP请求获取页面内容
使用requests库发送GET请求,获取题库网页的HTML内容。
```python
url = "http://www.example.com" # 替换为题库网址
response = requests.get(url)
```
步骤三:解析页面内容
使用BeautifulSoup库解析HTML内容,提取出题目等相关信息。
```python
soup = BeautifulSoup(response.text, 'html.parser')
question = soup.find('div', class_='question').text
options = soup.find_all('div', class_='option')
answers = soup.find_all('div', class_='answer')
```
步骤四:保存题目及答案信息
将题目和答案信息保存到本地文件或数据库中。
```python
with open('题库.txt', 'w', encoding='utf-8') as file:
file.write(question + '\n')
for i, option in enumerate(options):
file.write(f'{chr(i + 65)}. {option.text}\n')
for i, answer in enumerate(answers):
file.write(f'{chr(i + 65)}. {answer.text}\n')
```
以上是一个简单的Python爬取计算机二级WPS Office题库的代码示例,具体实现方式还可以根据题库网页的具体结构和需求进行调整和完善。
相关推荐
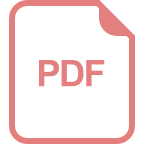
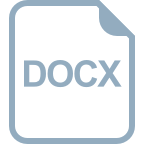
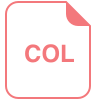
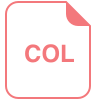
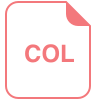
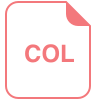
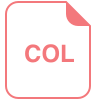









