设计一个测试用例,测试输出结果
时间: 2024-09-19 19:01:05 浏览: 38
为了设计一个测试用例,我们需要考虑以下几个步骤:
1. 确定目标:首先明确你要测试的是`groupAnagrams`函数的行为,特别是它应该能把字符串列表按照字母异位词分组。
2. 规定输入:选择不同的输入数据,涵盖各种可能的情况。例如:
- **正常情况**:包含完全相同的字符串,如`["abc", "cba", "abcc"]`
- **不同长度字符串**:包含长度不等的字符串,如`["a", "aa", "aaa", "ab", "ac"]`
- **空白和特殊字符**:包含包含空格、标点或其他非字母字符的字符串,如`["hello world", "world hello"]`
- **空输入**:验证函数能否正确处理空列表,如`[]`
- **混合输入**:混合包含相同字母异位词和不同字母异位词的字符串列表
3. 预期输出:针对上述输入,预期函数应返回的结果是什么样子。比如:
- 对于正常情况,应该返回[[“abc”, “cba”], [“abcc”]]
- 对于不同长度,可能返回[[“a”, “aa”], [“aaa”], ["ab", "ac"]]
- 含有空格和特殊字符的,可能返回[[“hello world”, “world hello”]]
4. 编写测试代码:在Python中,你可以使用unittest模坓编写这样的测试用例,如下所示:
```python
import unittest
from your_solution import groupAnagrams # 假设你的函数在此处
class TestGroupAnagrams(unittest.TestCase):
def test_same_string(self):
input_list = ["abc", "cba", "abcc"]
expected_output = [["abc", "cba"], ["abcc"]]
self.assertEqual(groupAnagrams(input_list), expected_output)
def test_diff_lengths(self):
input_list = ["a", "aa", "aaa", "ab", "ac"]
expected_output = [["a", "aa", "aaa"], ["ab", "ac"]]
self.assertEqual(groupAnagrams(input_list), expected_output)
def test_with_spaces(self):
input_list = ["hello world", "world hello"]
expected_output = [["hello world", "world hello"]]
self.assertEqual(groupAnagrams(input_list), expected_output)
def test_empty_input(self):
input_list = []
expected_output = []
self.assertEqual(groupAnagrams(input_list), expected_output)
def test_mixed_strings(self):
input_list = ["apple", "pale", "leap", "cape"]
expected_output = [["apple", "pale", "leap"], ["cape"]]
self.assertEqual(groupAnagrams(input_list), expected_output)
if __name__ == '__main__':
unittest.main()
```
5. 运行测试:运行测试用例,确保函数的行为符合预期。
阅读全文
相关推荐
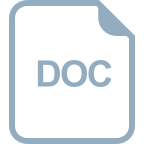
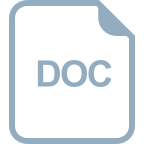
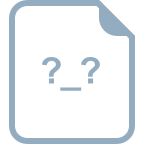
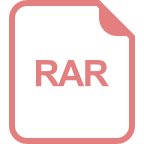
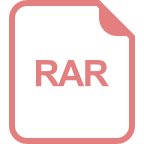
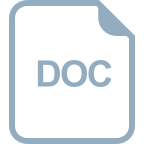
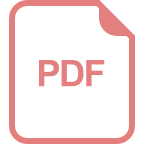
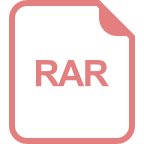
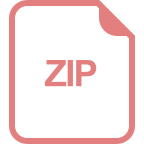
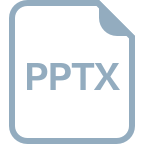
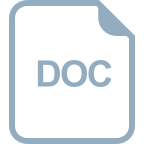
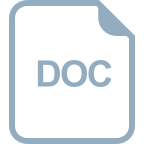
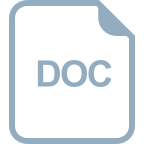
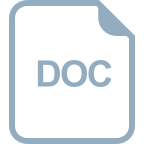
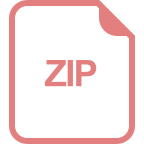
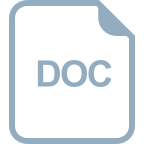
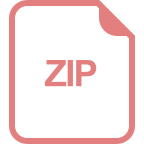